Summary: This page describes the method to creates a pulldown menu object. |
Relevant major classes of Java SE: java.awt.*, java.awt.event.*, javax.swing.*
|
Classes on this page: ButtonOfPulldownMenu, PulldownMenuAction, JMenuEx |
1. Overview
Creates a special type of pulldown menu with the hierarchy of JMenuBar - JMenu - JMenuItem. The pulldown menu can be added on the toolbar
like a button and it looks like a metallic button by painting on the JMenu
object (JMenuEx).
2. Class ButtonOfPulldownMenu
return=>page top
public class ButtonOfPulldownMenu extends JMenuBar
Field
|
Description
|
menu
|
JMenu menu
The JMenu object
|
menuAction
|
PulldownMenuAction menuAction=new PulldownMenuAction()
The ActionListener to be added to the menu.
|
Method
|
Description
|
Constructor
|
public ButtonOfPulldownMenu(String commandName, String tip)
Creates a button with the specified text (commandName) on the button.
Parameters:
commandName - The command name.
tip - The text to be displayed in a tool tip.
Processing:
Calls the following methods.
this.setLayout(new FlowLayout(FlowLayout.LEADING, 0, 0));
this.setName(commandName);
this.setOpaque(false);
this.menu=new JMenuEx();
this.add(this.menu);
this.menu.setName(commandName);
this.menu.setActionCommand(commandName);
this.menu.addActionListener(this.menuAction);
this.menu.setText(commandName);
this.menu.setToolTipText(tip);
this.setStandardButtonStyle();
|
Constructor
|
public ButtonOfPulldownMenu(String commandName, ImageIcon imageIcon, String
tip)
Creates a button with the specified ImageIcon on the button. The text (commandName)
is not displayed on the button.
Parameters:
commandName - The command name.
imageIcon - The imageIcon to be displayed on the button.
tip - The text to be displayed in a tool tip.
Processing:
Calls the following methods.
this.setLayout(new FlowLayout(FlowLayout.LEADING, 0, 0));
this.setName(commandName);
this.setOpaque(false);
this.menu=new JMenu();
this.add(this.menu);
this.menu.setName(commandName);
this.menu.setActionCommand(commandName);
this.menu.addActionListener(this.menuAction);
if(imageIcon!=null) this.menu.setIcon(imageIcon);
this.menu.setToolTipText(tip);
this.setStandardButtonStyle();
|
setStandardButtonStyle
|
public void setStandardButtonStyle()
Calls the following methods.
this.menu.setFont(MenuConstants.MenuFont);
Border raisedBorder = new BevelBorder(BevelBorder.RAISED);
this.menu.setBorder(raisedBorder);
this.menu.setIconTextGap(0);
this.menu.setOpaque(true);
Color backGround=new Color(0xDDE8F3);
this.menu.setBackground(backGround);
this.menu.setForeground(Color.black);
: If the menu field is assigned with the JMenuEx object in the constructor, then almost all of these settings are not referred.
|
setSelected
|
public void setSelected(boolean selected)
Sets the parameter to the menu.
|
isSelected
|
public boolean isSelected()
Returns if the menu is selected.
|
setMenuItems
|
public void setMenuItems(String[] menuItemNames, ImageIcon[] imageIcons,
String[] accelerators)
Sets the MenuItem objects to the menu. The ImageIcons[i] and the menuItemNames[i] are displayed on the
i-th MenuItem.
Paremeters:
menuItemNames - The array of the strings representing the names of the MenuItem objects.
imageIcons - The array of the ImageIcon objects to be displayed on the MenuItem
objects.
accelerators - The array of the strings representing the accelerators on the MenuItem
objects.
Processing:
(1) Calculates the maximum width of the menuItemNames by the getTextLayoutSize method.
(2) Creates MenuItem objects and adds them to the menu.
The label of the menuItemName[i] is add on the left side of the MenuItem[i] and the label of the
imageIcon[i] is add on the right side of the MenuItem[i]. The label width of the menuItemName[i] is determined by the result of step (1).
(3) Sets the action command and other attributes to the MenuItem[i]
as follow.
menuItem.setPreferredSize(new Dimension(menuItemWidth, menuItemHeight));
menuItem.setActionCommand(menuItemNames[i]);
menuItem.addActionListener(this.menuAction);
menuItem.setName(menuItemNames[i]);
|
getTextLayoutSize
|
private Dimension getTextLayoutSize(String text, Font font)
Returns the size of the text on a MenuItem object.
Creates the TextLayout object using the text, font and a new FontRenderContext
object, and gets the size of the text by the getAdvance, getAscent and
getDescent methods.
|
setSelectedMenuItem
|
public void setSelectedMenuItem(String menuItemName)
Paremeters:
menuItemName - The names of the MenuItem object
Processing:
This method changes the appearance of the selected MenuItem as the figre
below (dash item).
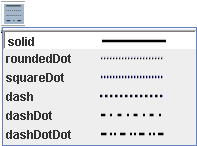
The following set up methods are called.
Border loweredBorder = new BevelBorder(BevelBorder.LOWERED);
menuItem.setBackground(Color.WHITE);
menuItem.setBorder(loweredBorder);
|
getMenuItem
|
public JMenuItem getMenuItem(JMenu menu, String menuItemName)
Parameters:
menu - JMenu object.
menuItemName - The name of the MenuItem object.
Returns:
The JMenuItem or JCheckBoxMenuItem object which is added to the menu.
Processing:
The Component objects under the menu can be got by the JMenu.getMenuComponents
method. The Command.compareStrings method is used to compare the specified menuItemName and the name of Component object.
|
createPageButton
(static)
|
public static ButtonOfPulldownMenu createPageButton(int width)
Parameters:
width - The width of the button.
Processing:
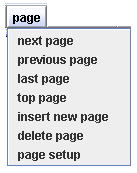
Creates the pulldown menu for the page setup. The settings are the follow.
String commandName=Command.getCommandLowerString(Command.PAGE);
String tip="page";
String[] menuItemNames={"next page", "previous page",
"last page", "top page",
"insert new
page", "delete page", "page setup"};
String[] imageName={"", "", "", "",
"", "", ""};
|
createEditButton
(static) |
public static ButtonOfPulldownMenu createEditButton(int width)
Parameters:
width - The width of the button.
Processing:
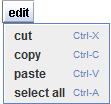
Creates the pulldown menu for the edit command. The settings are the follow.
String commandName=Command.getCommandLowerString(Command.EDIT);
String[] menuItemNames={"cut", "copy", "paste",
"select all"};
String[] imageName={"", "", "", "",
""};
String[] accelerators={"ctrl X", "ctrl C", "ctrl
V", "ctrl A"};
|
createShapeUtilButton
(static) |
public static ButtonOfPulldownMenu createShapeUtilButton(int width)
Parameters:
width - The width of the button.
Processing:
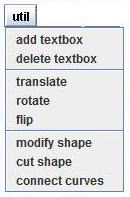
Creates the pulldown menu for the shape utility command. The settings
are the follow.
String commandName=Command.getCommandLowerString(Command.UTIL);
String[] menuItemNames={"add text box", "translate",
"rotate", "flip",
"modify
shape", "cut shape", "connect curves"};
String[] imageName={"", "", "", "",
"", "",""};
|
createSettingButton
(static) |
public static ButtonOfPulldownMenu createSettingButton(int width)
Parameters:
width - The width of the button.
Processing:
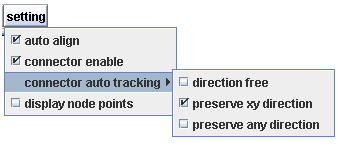
Creates the pulldown menu for the Setting command. This method doesn't
use the setMenuItems method. So creates each MenuItem object or JCheckBoxMenuItem object and
adds to the JMenu. See the source code for the details.
|
createTextAlignButton
(static)
|
public static ButtonOfPulldownMenu createTextAlignButton()
Processing:
Creates the pulldown menu for the text align command. The settings
are the follow.
String commandName=Command.getCommandLowerString(Command.TEXT_ALIGN);
String buttonImage="text_align_left32T.png";
String[] menuItemNames={"text align left", "text align center",
"text align right"};
String[] imageName={"text_align_left32T.png", "text_align_center32T.png",
"text_align_right32T.png"};
|
createFillColorChooser
Button
(static)
|
public static ButtonOfPulldownMenu createFillColorChooserButton(boolean
icon)
Parameters:
icon - If true, displays the icon on the button, otherwise displays the command name on the button
Processing:

Creates the pulldown menu for the fill color command. This method
doesn't use the setMenuItems method. So creates each MenuItem object and adds to the JMenu. See the
source code for the details.
|
createLineColorChooser
Button
(static)
|
public static ButtonOfPulldownMenu createLineColorChooserButton(boolean
icon)
Parameters:
icon - If true, displays the icon on the button, otherwise displays
the command name on the button
Processing:

Creates the pulldown menu for the line color command. This method
doesn't use the setMenuItems method. So creates each MenuItem object and adds to the JMenu. See the
source code for the details.
|
createLineWidthButton |
public static ButtonOfPulldownMenu createLineWidthButton(boolean icon)
Parameters:
icon - If true, displays the icon on the button, otherwise displays
the command name on the button
Processing:
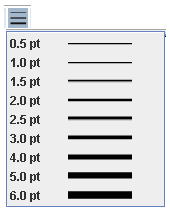
Creates the pulldown menu for the line width command. The settings
are the follow.
String commandName=Command.getCommandLowerString(Command.LINE_WIDTH);
String tip="line width";
String[] imageName
={"line0.5pt.png", "line1.0pt.png", "line1.5pt.png",
"line2.0pt.png", "line2.5pt.png",
"line3.0pt.png", "line4.0pt.png", "line5.0pt.png",
"line6.0pt.png"};
String[] menuItemNames
={"0.5 pt","1.0 pt", "1.5 pt", "2.0
pt", "2.5 pt", "3.0 pt", "4.0 pt", "5.0
pt", "6.0 pt"};
|
createLineStrokeButton |
public static ButtonOfPulldownMenu createLineStrokeButton(boolean icon)
Parameters:
icon - If true, displays the icon on the button, otherwise displays
the command name on the button
Processing:
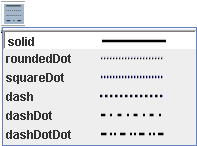
Creates the pulldown menu for the line stroke command. The settings
are the follow.
String commandName=Command.getCommandLowerString(Command.LINE_STROKE);
String tip="line stroke";
String[] imageName
={"dashed_style_solid.png", "dashed_style_roundedDot.png",
"dashed_style_squareDot.png",
"dashed_style_dash.png", "dashed_style_dashDot.png",
"dashed_style_dashDotDot.png"};
String[] menuItemNames
={"solid", "roundedDot", "squareDot", "dash",
"dashDot", "dashDotDot"};
|
createArrowStyleButton |
public static ButtonOfPulldownMenu createArrowStyleButton(boolean icon)
Parameters:
icon - If true, displays the icon on the button, otherwise displays the command name on the button
Processing:
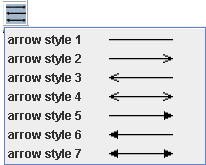
Creates the pulldown menu for the arrow style command. The settings
are the follow.
String commandName=Command.getCommandLowerString(Command.ARROW_STYLE);
String tip="arrow style";
String[] imageName
={"arrow_style1.png", "arrow_style2.png", "arrow_style3.png",
"arrow_style4.png",
"arrow_style5.png", "arrow_style6.png", "arrow_style7.png"};
String[] menuItemNames
={"arrow style 1", "arrow style 2", "arrow style
3", "arrow style 4",
"arrow style 5", "arrow style 6", "arrow style
7"};
|
createZorderButton |
public static ButtonOfPulldownMenu createZorderButton(boolean icon)
Parameters:
icon - If true, displays the icon on the button, otherwise displays
the command name on the button
Processing:
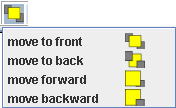
Creates the pulldown menu for the Z order command. The settings are
the follow.
String commandName=Command.getCommandLowerString(Command.Z_ORDER);
String tip="depth position";
String[] menuItemNames={"move to front", "move to back",
"move forward",
"move backward"};
String[] imageName={"move_to_front32T.png", "move_to_back32T.png",
"move_forward32T.png", "move_backward32T.png"};
|
createAlignButton |
public static ButtonOfPulldownMenu createAlignButton(boolean icon)
Parameters:
icon - If true, displays the icon on the button, otherwise displays
the command name on the button
Processing:
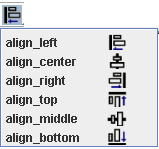
Creates the pulldown menu for the shape align command. The settings
are the follow.
String commandName=Command.getCommandLowerString(Command.ALIGN);
String tip="align";
String[] menuItemNames={"align_left", "align_center",
"align_right",
"align_top", "align_middle", "align_bottom"};
String[] imageName={"align_left32T.png", "align_center32T.png",
"align_right32T.png", "align_top32T.png",
"align_middle32T.png", "align_bottom32T.png"};
|
createTestButton |
public static ButtonOfPulldownMenu createTestButton(int width)
Parameters:
width - The width of the button.
Processing:
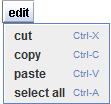
Creates the pulldown menu for the Test command. This method doesn't use
the setMenuItems method. So creates each MenuItem object and adds to the JMenu. See the
source code for the details.
|
3. PulldownMenuAction
return=>page top
class PulldownMenuAction extends AbstractAction
Field
|
Description
|
buttonOfPulldownMenu
|
ButtonOfPulldownMenu buttonOfPulldownMenu
The ButtonOfPulldownMenu object which created this object.
|
Method
|
Description
|
Constructor
|
PulldownMenuAction(ButtonOfPulldownMenu buttonOfPulldownMenu)
Sets the parameter to the field.
|
actionPerformed
|
public void actionPerformed(ActionEvent e)
Sets the commandId and command parameters (params) as follow and calls the exec method of the ExecCommand.
∙ commandId
int commandId
=Command.getCommandId(this.buttonOfPulldownMenu.menu.getActionCommand());
∙ Command parameters:
params[0]=e.getActionCommand();
params[1]=e;
|
4. JMenuEx
return=>page top
class JMenuEx extends JMenu
This object provides a JMenu object with the appearance of a metallic
button.
Field
|
Description
|
baseColor |
Color baseColor=new Color(0xCDD8F3);
The color at the lower part of the button. |
Method
|
Description
|
paintComponent
|
public void paintComponent(Graphics g)
Paints the JMenu component and display the text retrieved by the getText
method.
|
|