1.Class ButtonOfDebug
return=>page top
1.1 The menu and menu items of the debug command
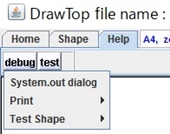 |
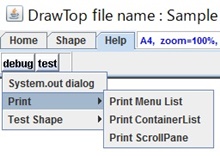 |
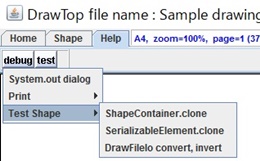 |
(a) The menu of the debug command |
(b) The menu items of the Print command |
(c) The menu items of the Test Shape command |
Figure 1. The debug command
1.2 API
public class ButtonOfDebug extends JMenuBar implements ActionListener
:
ButtonOfDebug object is created by the DrawMenu.createDebugTestGroup method
and is added to the ToolBar panel.
Field
|
Description
|
menu
|
JMenu menu
Set JMenu object.
ButtonOfDebug is an extended class of JMenuBar and its display is the debug button in
Figure 1 (a).
The menu object is added (add) to ButtonOfDebug and displayed under the debug button
as shown in Fig. 1 (a).
|
Method
|
Description
|
Constructor
|
public ButtonOfDebug(String commandName, boolean setText, ImageIcon imageIcon,
String tip)
Creates a button with the specified ImageIcon on the button.
Parameters:
commandName - The command name.
setText - If true, displays the command name on the button.
imageIcon - The imageIcon to be displayed on the button.
tip - The text to be displayed in a tool tip.
|
setStandardButton Style
|
public void setStandardButtonStyle()
Calls the following methods.
this.menu.setFont(MenuConstants.MenuFont);
Border raisedBorder = new BevelBorder(BevelBorder.RAISED);
this.menu.setBorder(raisedBorder);
this.menu.setIconTextGap(0);
this.menu.setOpaque(true);
Color backGround=new Color(0xDDE8F3);
this.menu.setBackground(backGround);
this.menu.setForeground(Color.black);
|
setSelected |
public void setSelected(boolean selected)
Sets the parameter to the menu.
|
isSelected
|
public boolean isSelected()
Returns if the menu is selected.
|
createDebugButton (static) |
public static ButtonOfDebug createDebugButton(int width)
Parameters:
width - The width of the button.
Processing:
The settings are the follow.
ButtonOfDebug menuButton=new ButtonOfDebug("debug", true, null, commandName);
String[] menuItemNames={"System.out dialog","print menulist"};
|
actionPerformed
|
public void actionPerformed(ActionEvent e)
Executes the processing according to the menu item.
|
2. Class DialogOfSystemOut
return=>page top
2.1 Display of the SystemOutDialog dialog
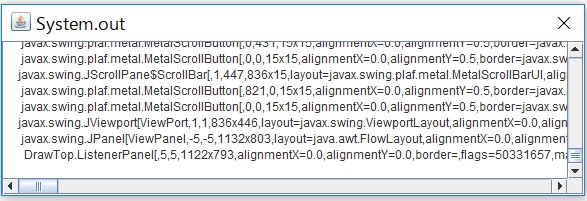
Figure 2. SystemOutDialog
Displays the dialog of the standard output and error output.
2.2 API
public class DialogOfSystemOut implements WindowListener
Method
|
Description
|
Constructor
|
public DialogOfPageSetup()
Sets the following.
super(ObjectTable.getDrawMain(), "System.out");
this.setName("DialogOfSystemOut");
this.addWindowListener(this);
|
showDialog
|
public void showDialog()
∙ If this dialog is already opened, this method does nothing.
Whether the dialog is opened or not can be checked by the by the
getMenuComponent of the
menuUtil.
∙ Creates a new JTextArea object and a new JScrollPane object , and then
adds the new JTextArea to the new JScrollPane.
∙ Creates a new TextAreaOutputStream object and sets it to the standard output stream.
TextAreaOutputStream outputStream=new TextAreaOutputStream(textArea);
System.setOut(new PrintStream(outputStream));
System.setErr(new PrintStream(outputStream));
∙ Shows the dialog by calling this.setVisible(true).
∙ Registers this object to the MenuComponentList the
setMenuComponent.
|
closeDialog |
private void closeDialog()
Sets this dialog invisible and removes this from the MenuComponentList.
Restores the standard output stream.
FileOutputStream fileOut=new FileOutputStream(FileDescriptor.out);
PrintStream out=new PrintStream(new BufferedOutputStream(fileOut), true);
System.setOut(out);
System.setErr(out);
|
windowClosing
|
public void windowClosing(WindowEvent e)
Calls the closeDialog method.
|
3. Class TextAreaOutputStream
return=>page top
public class TextAreaOutputStream extends OutputStream
Field
|
Description
|
textArea
|
JTextArea textArea
JTextArea object.
|
Method
|
Description
|
Constructor
|
public TextAreaOutputStream(JTextArea textArea)
Sets the parameter to the field.
|
write
|
public void write(int i)
this.textArea.append(Character.toString((char)i));
|
write
|
public void write(char[] buf, int off, int len)
String s = new String(buf, off, len);
this.textArea.append(s);
|
|