1. Overview
1.1 The commands using the ButtonOfColorChooser
(1) fill color:
The "fill color" menu item of the "fill color"
button uses the ButtonOfColorChooser (Figure 1).
(2) line color:
The "line color" menu item of the "line color"
button uses the ButtonOfColorChooser (Figure 1).
(3) font color
Click the font button ( ), then the "font menu" dialog appears below the font button.
The button uses the ButtonOfColorChooser.
1.2 The commands using the ButtonOfColorChooser
(1) The operation to click the ButtonOfColorChooser are three ways:
・Selecting the "fill color" menu item of the "fill color"
button.
・Selecting the "line color" menu item of the "line color"
button.
・Clicking the button.
(2) The processing after clicking the ButtonOfColorChooser. >
The actionPerformed method of the ColorChooserAction
object is called to perform the action of the ButtonOfColorChooser.
public void actionPerformed(ActionEvent e) {
this.commandName=e.getActionCommand();
............................
CustomColorChooserDialog dialog=ObjectTable.getCustomColorChooserDialog();
String title="";
if(this.commandName.equals("fill color")) title="
Command: fill color";
if(this.commandName.equals("line color")) title="
Command: line color";
if(this.commandName.equals("font color")) title="
Command: font color";
dialog.commandLabel.setText(title);
dialog.removeColorSelectionListener();
dialog.addColorSelectionListener(this);
dialog.showDialog(this.commandName);
}
Retrieves the command name by e.getActionCommand(). The command name is one of the "fill color", "line color" or
"font color". Next, gets the CustomColorChooserDialog object
from the ObjectTable and performs the following processing:
Step1 Specifies the title which is displayed in the
CustomColorChooserDialog such as "Command: fill color" etc.
The title is the string on the black color panel (Figure_(b)).
Step2 Resets and sets the ColorSelectionListener.
When the "OK" button is clicked on the CustomColorChooserDialog,
the ColorChooserAction object which implements the
ColorSelectionListener interface receives the
ColorSelectionEvent by the colorSelected method.
step3 Displays the CustomColorChooserDialog by calling the showDialog method
with the parameter of the command name.
:
About the CustomColorChooserDialog object.
Three ButtonOfColorChooser objects are created for the three
commands of "fill color", "line color" and "font
color". However, the CustomColorChooserDialog object is created only
one and it is used in common for the three commands.
The reason that the CustomColorChooserDialog object is only one is something
as follows:
Even if the three commands such as "fill color", "line color" or "font color" are called in any order, the following two data must be kept without change..
(a) The information of the colors which is imported into the CustomColorChooserDialog.
(b) The display location of the CustomColorChooserDialog.
If the three ButtonOfColorChooser objects are created, another common object
will be needed to keep the data (a) and (b). It is cumbersome.
1.3 The processing after clicking the OK button of the CustomColorChooserDialog
The processing after clicking the OK button of the CustomColorChooserDialog
is as follows:
The colorSelected method of the ColorChooserAction object is called.
The code of the colorSelected method is as follows:
public void colorSelected(ColorSelectionEvent event) {
Color selectedColor = event.getColor();
if(selectedColor==null) return;
Object[] args = new Object[1];
args[0] = selectedColor;
int commandId = Command.getCommandId(this.commandName);
if (commandId < 0) {
System.err.println("*** Error ColorChooserAction: specified
command not found;" + commandName);
return;
}
Command command = new Command(commandId, Command.callFromMenu, args);
ExecCommand execCommand = ObjectTable.getExecCommand("ColorChooserAction");
execCommand.exec(command);
}
Retrieves the color from the ColorSelectionEvent, creates the command which is one of "fill color", "line
color" or "font color" and executes the command
by calling the ExecCommand.exec method.
2. Class ButtonOfColorChooser
return=>page top
public class ButtonOfColorChooser extends JButton
Field
|
Description
|
action |
ColorChooserAction action=new ColorChooserAction(this);
The ActionListener of this button. |
currentColor
|
Color currentColor
The selected color by this color chooser.
|
Method
|
Description
|
Constructor
|
ColorChooserButton(String commandName, String tip)
Creates a button with the specified text (commandName) on the button.
Parameters:
commandName - The command name.
tip - The text to be displayed in a tool tip.
Processing:
Calls the following methods.
this.setActionCommand(commandName);
this.addActionListener(this.action);
this.setName(commandName);
this.setText(commandName);
this.setToolTipText(tip);
this.setStandardButtonStyle();
|
Constructor
|
public ButtonOfColorChooser(String commandName, boolean setText, ImageIcon
imageIcon, String tip)
Parameters:
commandName - The command name.
setText - If true, displays the commandName on the button.
imageIcon - The ImageIcon to be displayed on the button.
tip - The text to be displayed in a tool tip.
Processing:
Calls the following methods.
super(imageIcon);
this.setActionCommand(commandName);
this.addActionListener(this.action);
this.setName(commandName);
if(setText) setText(commandName);
this.setToolTipText(tip);
this.setStandardButtonStyle();
|
setStandardButtonStyle
|
public void setStandardButtonStyle()
Calls the following methods.
Border raisedBorder = new BevelBorder(BevelBorder.RAISED);
this.setBorder(raisedBorder);
this.setIconTextGap(0);
this.setHorizontalTextPosition(SwingConstants.CENTER );
this.setVerticalTextPosition(SwingConstants.BOTTOM );
this.setHorizontalAlignment(CENTER);
this.setFont(MenuConstants.MenuFont);
this.setBackground(null);
this.setForeground(Color.BLACK);
this.setOpaque(true);
|
getCurrentColor
|
public Color getCurrentColor()
Returns the currentColor.
|
setCurrentColor
|
public void setCurrentColor(Color color)
Sets the parameter to the currentColor.
|
createColorChooser
Button
|
public static JButton createColorChooserButton(String commandName, int
width, String tip)
Parameters:
commandName - The command name.
width - The button width.
If the width<0, then the button width is determined by using the TextLayout.
tip - The text to be displayed in a tool tip.
Processing:
Creates a new button object by the first Constructor.
|
createColorChooser
Button
|
public static JButton createColorChooserButton(String commandName, boolean
setText, String imageName, String tip)
Parameters:
commandName - The command name.
setText - If true, displays the commandName at the right side of the ImageIcon on the button.
imageName - The image name if the ImageIcon.
The imageName doesn't need to include the file path.
tip - The text to be displayed in a tool tip.
Processing:
Creates a new button object by the second Constructor. The button displays the image specified by the imageName on the button.
|
3. ColorChooserAction
return=>page top
class ColorChooserActionn extends AbstractAction
Method
|
Description
|
actionPerformed
|
public void actionPerformed(ActionEvent e)
∙ Shows the CustomColorChooserDialog object by the showDialog method.
The CustomColorChooserDialog object is created by the DrawMain
and is registered to the ObjectTable.
∙ Sets the command name to the CustomColorChooserDialog.
The command names are "fill color", "line color" or
"font color". One of these commands displays the CustomColorChooserDialog.
∙ Sets the ColorSelectionListener to thisobject.
Before setting, all registered ColorSelectionListeners must be removed.
dialog.removeColorSelectionListener();
dialog.addColorSelectionListener(this);
dialog.showDialog();
|
colorSelected |
public void colorSelected(ColorSelectionEvent event)
This method is defined by the ColorSelectionListener.
∙ This method is called when the "OK button" of the CustomColorChooserDialog is pressed and gets the color in the following manner.
Color selectedColor = event.getColor();
∙ Generates a Command object according to the action command and calls the exec method of the ExecCommand with the Command object.
(a)commandId
int commandId
=Command.getCommandId
(this.buttonOfPulldownMenu.menu.getActionCommand());
(b)parameter
params[0]=selectedColor;
|
4. CustomColorChooserDialog class
return=>page top
public class CustomColorChooserDialog extends JDialog implements ActionListener,
ComponentListener, WindowListener, SelectionListener
4.1 Summary
ColorChooser dialog which is used instead of the javax.swing.JColorChooser.
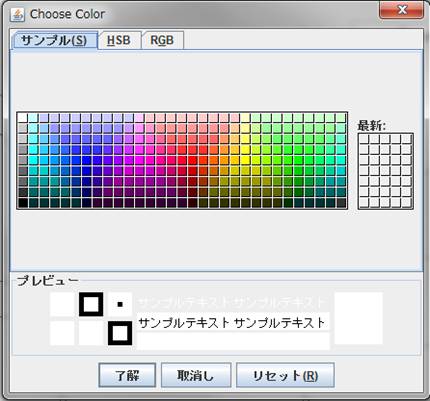
|
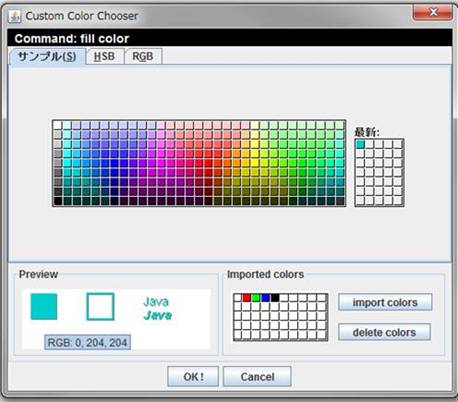
|
Figure_(a) Dialog of the JColorChooser |
Figure_(b) Dialog of the CustomColorChooserDialog |
The features of CustomColorChooserDialog
Feature
|
Description
|
(1)"import colors" button
|
=>
Figure_(b)
• Initial state
No color is imported to the "imported colors" panel just after
the Drawtop application is started. However, the white, black, red, green
and blue colors are shown on the panel for reference.The "Importing
colors" procedure is carried out in the following manner.
• Importing colors
Select shapes on the drawing panel (canvas) and click the "imported
colors" button, the fill colors, the line colors and the font colors
of the selected shapes will be imported to "imported colors" panel.
• Specifying a color
If you specify a color to the selected shapes or the selected texts, you
can select a color on the upper JColor chooser panel of the Figure_(b)
or the "Imported colors panel" of the Figure_(b). The selected
color will be displayed immediately on the preview panel. If the selected
color is good and you click the "OK!" button,
then the selected shapes or the texts change to the color.
|
(2)"delete colors" button |
If you select colors by clicking on the "imported colors" panel
and press the "delete colors" button, then the selected colors
will be deleted from the "imported colors" panel. Multiple
colors can be selected by clicking a mouse with holding down the Shift
or Ctrl key.
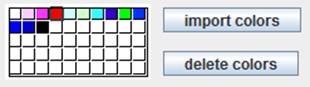
: The selected colors are displayed with the dark gray frames. |
(3)Receiving the selected color from the CustomColorChooserDialog |
=>1.3
The class which receives the selected color must implement ColorSelectionListener interface and the colorSelected method as follows.
public void colorSelected(ColorSelectionEvent event) {
Color color = event.getColor();
}
:
The ColorChooserAction object receives the selected color in this application.
|
4.2 API
Field
|
Description
|
commandName |
String commandName="";
This is the name which is displayed on the upper black panel in the dialog
( Figurer(b) ). One of the "fill color", "line color" or "font
color" is set to this field. |
commandLabel |
JLabel commandLabel;
The upper black panel in the dialog ( Figurer(b)). |
colorChooser
|
JColorChooser colorChooser
JcolorChooser panel object which is the upper side of the dialog in the
the Figurer(b).
|
previewPanel |
PreviewPanel previewPanel
Preview panel object which is the left lower side of the dialog in
the the figurer(b).
|
importedColorsPanel |
ImportedColorsPanel importedColorsPanel
"Imported colors" panel object which is the right lower
side of the dialog in the the figurer(b).
|
dialogLocation |
Point dialogLocation=new Point(100, 100);
The dialog position on the frame of DrawMain.
|
okButton |
JButton okButton
"OK button" object.
|
cancelButtonl |
JButton cancelButtonl
"Cancel button" object.
|
importButton |
JButton importButton
"Import button" object on the importedColorsPanel.
|
deleteButton |
JButton deleteButton
"Delete button" object on the importedColorsPanel.
|
listenerList |
protected EventListenerList listenerList = new EventListenerList();
The list storing the ColorSelectionListeners.
|
Method
|
Description
|
Constructor
|
public CustomColorChooserDialog(JFrame frame)
Parameters:
frame - the parent frame of this object.
Processing:
Initialize this dialog by calling initializeDialog method.
|
initializeDialog |
public void initializeDialog()
Initialize this dialog.
• Create the colorChooser, importedColorsPanel and previewPanel objects
and set them to the field variables.
• Create the button objects and set them the field variables. |
showDialog |
public void showDialog(String commandName)
• Display this dialog.
• Sets WindowListener to this dialog.
: Initial setting to the colorChooserand importedColorsPanel
this.getColorChooser().setColor(Color.WHITE);
this.getColorChooser().setColor(Color.BLACK);
Color[] colors={Color.WHITE, Color.BLACK, Color.RED, Color.GREEN, Color.BLUE};
this.getImportedColorsPanel().setImportedColors(colors);
|
getCommandName |
protected String getCommandName()
Returns the commandName. |
getColorChooser |
public JColorChooser getColorChooser()
Returns the colorChooser.
|
getPreviewPanel |
protected PreviewPanel getPreviewPanel()
Returns the previewPanel.
|
getImportedColorsPanel |
protected ImportedColorsPanel getImportedColorsPanel()
Returns the importedColorsPanel.
|
setPreviewColor |
public void setPreviewColor(Color color, String command)
Sets a color to the previewPanel.
|
getPreviewColor |
public Color getPreviewColor(String command)
Returns the color of the previewPanel.
|
actionPerformed |
public void actionPerformed(ActionEvent e)
Handle the action processing of the "OK
button", "Cancel button", "Import colors check
box" and "Delete button" .
: "Import colors check box" and "Delete button"
belong to the "Imported colors panel", so their action processing
may be handled in the ImportedColorsPanel object. However it is handled
here (in the CustomColorChooserDialog) for easy understanding of the codes
structures.
• OK button
Gets the color of the previewPanel and creates a
ColorSelectionEvent by the fireEvent method.
• Cancel button - calls closeDialog method.
• import button
Imports the colors of selected shapes on the canvas.
• Delete button
Calls the removeCurrentColors method of the importedColorsPanel.
|
importColors |
public void importColors()
Sets all the colors including font color of the selected shapes to the
importedColorsPanel. |
selected |
public void selected(SelectionEvent event)
The method defined by the SelectionListener.
=>(1)Import colors check box |
addColorSelection
Listener |
public void addColorSelectionListener(ColorSelectionListener listener)
Registers the objects which are interesting in receiving the ColorSelectionEvent.
=>(3)Receiving the selected color from the CustomColorChooserDialog
|
removeColorSelection
Listener |
public void removeColorSelectionListener(ColorSelectionListener listener)
Removes the objects which were registered by the above method.
|
removeColorSelection
Listener |
public void removeColorSelectionListener()
Removes all the objects which were registered by the addColorSelectionListener
method. |
fireEvent |
public void fireEvent(ColorSelectionEvent event)
Reports the ColorSelectionEvent to the objects registered by the addColorSelectionListener method. |
closeDialog |
protected void closeDialog()
Closes this dialog.
• Removes all objects from the listenerList
by calling the removeColorSelectionListener method.
• Removes this dialog from the SelectionLS by calling the
SelectionLS
removeSelectionListener method.
|
windowClosing |
public void windowClosing(WindowEvent e)
This method is defined by the WindowListener.
Calls the closeDialog method.。
|
componentMoved |
public void componentMoved(ComponentEvent e)
This method is defined by the ComponentListener.
Sets the current dialog location to the dialogLocation field. |
5. ColorSelectionListener interface
return=>page top
interface ColorSelectionListener extends EventListener
Method
|
Description
|
colorSelected
|
public void colorSelected(ColorSelectionEvent event)
The method which is called when a color selection event (ColorSelectionEvent) occurs.
|
6. ColorSelectionEvent class
return=>page top
Class ColorSelectionEvent
Field
|
Description
|
color
|
Color color
The color which is set to this event object.
|
Method
|
Description
|
Constructor
|
public ColorSelectionEvent(Object source, Color color)
|
getColor |
public Color getColor()
Returns the value of the color field. |
|