|
1. Source code download
=>
CustomColorChooser1.zip,
CustomColorChooser2.zip
The two zip files are corresponding to the test code (1) and
the test code (2) described below. They must be executed in different projects.
2. Features of CustomColorChooser
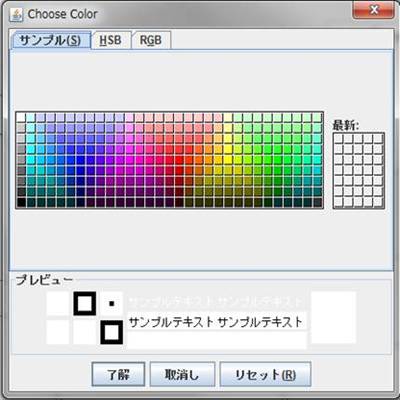
|
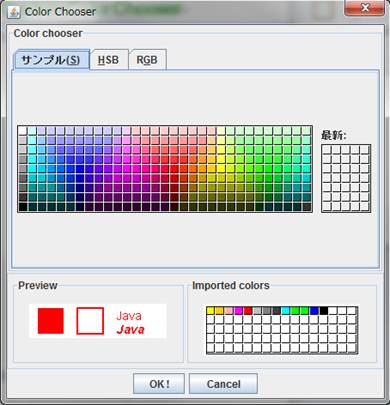
|
(a) Dialog of javax.swing.JColorChooser |
(b) Dialog of CustomColorChooserDialog |
Features
|
Description
|
(1)Importing colors
|
The purpose of a custom color chooser is to be able to reuse the colors
of drawings already designed. For this purpose we add an ability to
the current color chooser which ability can import colors from existing
drawings.
The "Imported colors" panel at the lower right of the Figure (b) is such an example.
As a method for importing colors, there may be several ways as
follows.
(a) Import all the colors used in the drawings file.
(b) Import all the colors used in a page of drawings file.
(c) Import the colors of selected shapes.
In this article, we will configure test codes aiming at the method (c).
:The colors that should be imported must be the outline colors of
shapes, the fill color of shapes and the text colors if the shapes have
texts.
|
(2)Reusing colors |
The following functions are required.
(a) If a color is clicked on the color palette of "Imported colors"
panel, the color must be displayed on the preview panel immediately.
(b) If a color is clicked on the color palette of the javax.swing.JColorChooser (the upper side of the figure (b) ), the color must be also displayed on a preview panel.
(c) If the OK button is pressed, the color on the preview panel must be
passed to the application that set the color to the selected shapes or
the selected text.
|
3. Test code (1)
❐ Key components
Component
|
Description
|
CustomColor Chooser1
|
public class CustomColorChooser1
The class implementing the main method. This object receives a selected
color from the CustomColorChooserDialog as follows.
Color color=CustomColorChooserDialog.showDialog(frame, colors);
The colors is an array of colors to be passed from the application, those colors are displayed in the "Imported colors" panel in the figure (b).
The above CustomColorChooserDialog.showDialog static method works completely like the JColorChooser.showDialog method.
|
CustomColor ChooserDialog |
class CustomColorChooserDialog extends JDialog implements ActionListener, ComponentListener
The dialog shown in figure (b).
(1) Set up the JColorChooser, PreviewPanel, ImportedColorsPanel, OK button
and Cancel button in this dialog.
this.colorChooser = new JColorChooser();
this.colorChooser.setBorder(BorderFactory.createTitledBorder("Color
chooser"));
this.colorChooser.setPreviewPanel(new JLabel("Preview", JLabel.CENTER));
The above third line sets a dummy to the PreviewPanel of the
JColorChooser. The new PreviewPanel is added to the CustomColorChooserDialog
(JDialog extended).
(2) Return of the showDialog method
return dialog.getPreviewColor();
The getPreviewColor method returns the color displayed in the Preview Panel.
In the case of the Figure (b), the method returns red.
|
PreviewPanel |
class PreviewPanel extends JComponent implements ChangeListener, ColorSelectionListener,
MouseListener
The new PreviewPanel which is different from the PreviewPanel attached to JColorChooser. This new PreviewPanel displays the color to the panel which was selected on the JColorChooser or the ImportedColorsPanel.
(1) Receiving the selected color from the JColorChooser
Register this object to the ColorSelectionModel of the JColorChooser
as a ChangeListener.
Add the following line to the Constructor of this class.
dialog.getColorChooser().getSelectionModel().addChangeListener(this);
:The dialog represents the CustomColorChooserDialog object.
(2) Receiving the selected color from the ImportedColorsPanel.
Register this object to the ImportedColorsPanel as a ColorSelectionListener by the addColorSelectionListener method of the ImportedColorsPanel.
Add the following line to the Constructor of this class.
dialog.getImportedColorsPanel().addColorSelectionListener(this);
|
ImportedColorsPanel |
class ImportedColorsPanel extends JComponent implements MouseListener
Receive an array of colors from an external application and display them
on the color palette on this panel.
A color on the color palette can be selected with a mouse click and the
selected color must be reported to the PreviewPanel. So the color selection
mechanism and color reporting mechanism to other object is required for
this panel.
(1) Receive an array of colors from the external application
Receive them by the following line ( => CustomColorChooser1)
Color color=CustomColorChooserDialog.showDialog(frame, colors);
The argument colors is the array of colors from an external application.
(2)Display the colors
The colors are displayed in the same way as that of SwatchPanel in the javax.swing.colorchooser.DefaultSwatchChooserPanel.java
(3) Select a color with a mouse
This class is implemented with the MouseListener in order to be able to select a color using the mousePressed method.
(4) Notification to the selected color to the PreviewPanel
Create a ColorSelectionEvent and notify a color selection event to other object with the ColorSelectionEvent
by the fireEvent method of this class.
For this reason, the addColorSelectionListener and the removeColorSelectionListener
methods are required in this object to register a ColorSelectionListener and remove it.
The recipient (in this case, the PreviewPanel) must implements the colorSelected method defined by the ColorSelectionListener to receive the selected color.
|
❐ColorSelectionListener
Item
|
Description
|
code
|
interface ColorSelectionListener extends EventListener {
public void colorSelected(ColorSelectionEvent event);
} |
implementation |
Implement to PreviewPanel. |
❐ColorSelectionEvent
Item
|
Description
|
code
|
class ColorSelectionEvent extends EventObject {
Color color=null;
public ColorSelectionEvent(Object source, Color color) {
super(source);
this.color=color;
}
public Color getColor(){
return this.color;
}
} |
❐SwatchPanel
javax.swing.colorchooser.DefaultSwatchChooserPanel.javaのSwatchPanel class
Display colors by the paintComponent method.
Item
|
Description
|
code
|
public void paintComponent(Graphics g) {
g.setColor(getBackground());
g.fillRect(0, 0, getWidth(), getHeight());
for (int row = 0; row < numSwatches.height; row++) {
for (int column = 0; column < numSwatches.width; column++)
{
g.setColor(getColorForCell(column, row));
int x;
if ((!this.getComponentOrientation().isLeftToRight())
&& (this instanceof RecentSwatchPanel))
{
x = (numSwatches.width - column - 1) * (swatchSize.width
+ gap.width);
} else {
x = column * (swatchSize.width + gap.width);
}
int y = row * (swatchSize.height + gap.height);
g.fillRect(x, y, swatchSize.width, swatchSize.height);
g.setColor(Color.black);
g.drawLine(x + swatchSize.width - 1, y, x + swatchSize.width
- 1,
y + swatchSize.height - 1);
g.drawLine(x, y + swatchSize.height - 1, x + swatchSize.width
- 1,
y + swatchSize.height - 1);
}
}
}
|
4. Test code (2)
❐Dialog
Item
|
Description
|
"Imported colors"
panel
|
In the CustomColorChooser1, an array of the colors imported from an
application is passed to the argument of the showDialog method as folloes.
Color color=CustomColorChooserDialog.showDialog(frame, colors);
Since this method may have little flexibility, we implement the "Import
colors" button which imports an array of colors into the palette of
the "Imported colors" panel, and the "delete colors"
button which deletes a imported color from the palette.
For importing an array of colors, we select shapes on the canvas and click the
"Import colors" button , then the colors of the selected shapes
will be imported.
However this is a test code and shape selection mechanism is not implemented,
so when the button is clicked, an array of colors prepared beforehand are
imported.
|
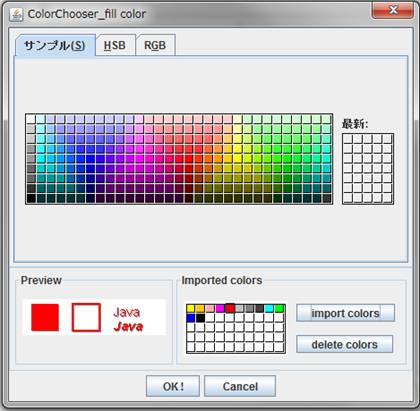
|
Figure (c) Dialog of the CustomColorChooserDialog |
❐ Changed points of the key components
Component
|
Description
|
CustomColor Chooser2
|
public class CustomColorChooser2 implements ColorSelectionListener
This object receives a selected color from the CustomColorChooserDialog
as follows.
In the Test code (1), the modal color-chooser dialog shown by the JColorChooser.showDialog
method blocks until the dialog is hidden.
=> (3)Receiving the selected color from javax.swing.JColorChooser
This method doesn't allow selection mechanism of shapes by clicking the mouse button
on the canvas. Therefor, we implement the ColorSelectionListener to an application (in this case, the application is CustomColorChooser2)
and the application receives the array of colors by the colorSelected method.
(2)Code
public static void main(String[] args)
..............................
CustomColorChooser2 chooser=new CustomColorChooser2();
CustomColorChooserDialog dialog=new CustomColorChooserDialog(frame);
dialog.addColorSelectionListener(chooser);
dialog.showDialog();
}
public void colorSelected(ColorSelectionEvent event) {
Color color = event.getColor();
System.out.println("** CustomColorChooser colorSelected event.getColor()="
+ event.getColor());
}
|
CustomColor ChooserDialog |
class CustomColorChooserDialog extends JDialog implements ActionListener
Additional parts to the Test code (1) are as follows.
(1) "Import colors" button and "delete colors" button
(2) actionPerformed method
In this method, the action processing for the events from the
"Import colors" button and "delete colors" button are
described.
|
PreviewPanel |
class PreviewPanel extends JComponent implements ChangeListener, ColorSelectionListener,
MouseListener
Same as the Test code (1) |
ImportedColorsPanel |
class ImportedColorsPanel extends JComponent implements MouseListener
Additional parts to the Test code (1) are as follows.
(1) Implement the method to import an array of colors when the "Import
colors" button is clicked.
public void setImportedColors(Color[] colors)
: Implement a color duplication check and color sorting functions to the
setImportedColors method.
(2) Implement a color selection method by a mouse click.
This method can be easily implemented using the MouseListener's methods.
In addition, implementing the following two functions will be better.
• Deselect a color which is already selected.
• Select multiple colors with holding down the Shift-key or Ctrl-key.
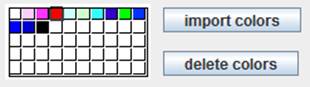
In addition, the dark gray frames will be displayed around the selected colors.
(3)Implement a method to delete selected colors from the color palette.
public void removeCurrentColors()
|
|