1. Class PageManager
public class PageManager
This class manages multiple pages and provides the methods
to insert a new page (PageData object),
delete the current page, change the current page to other page,
write page data to a file and read page data from a file.
The multiple pages and their order can be displayed in the
dialog (DialogOfPageLayout) as follow.
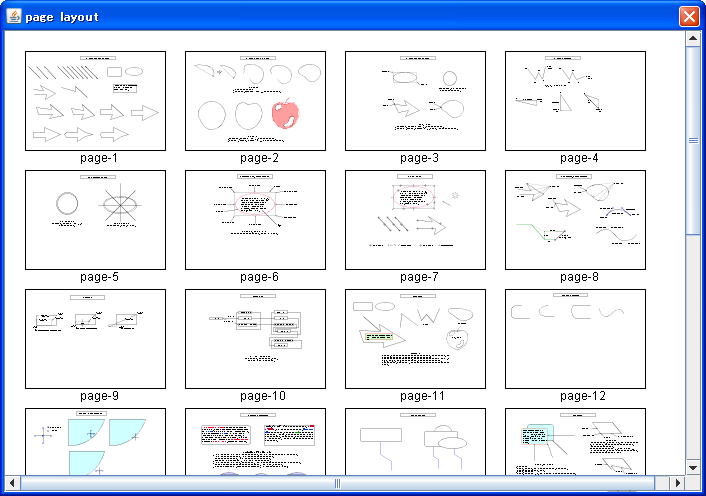
Figure 1. Page layout dialog
Field
|
Description
|
PageList
|
private ArrayList PageList
The ArrayList storing PageData objects corresponding to multiple pages.
=>Figure 2
|
CurrentPage
|
private int CurrentPage
The current page number being displayed on the screen (1,2,.....n).
|
versions
|
String[] versions
The array of the strings representing the versions of file format.
|
Method
|
Description
|
init
|
public void init()
Creates new ArrayList object for the PageList and calls the insertNewPage method.
|
setPageList
|
public void setPageList(ArrayList pageList)
Parameters:
pageList - The ArrayList object.
Processing:
Sets the pageList to the PageList field. This method is used in the readPageList method.
|
getPageList
|
public ArrayList getPageList()
Returns the PageList.
|
getCurrentPage
|
public int getCurrentPage()
Returns the CurrentPage.
|
getUndoDrawManager
|
public UndoDrawManager getUndoDrawManager()
Returns the UndoDrawManager object of the current page.
=>PageData
|
showTopPage
|
public void showTopPage()
Saves the current page to the
PageList by the saveCurrentPage
method and shows the top page by the showPage method.
This method is called by the ExecCommand.exec().
|
showLastPage
|
public void showLastPage()
Saves the current page to the PageList
by the saveCurrentPage method and
shows the last page by the showPage method.
This method is called by the ExecCommand.exec().
|
initApp
|
private void initApp()
∙ Deselcts all the shape elements on the screen.
∙ Deletes all the temporary shapes and texts on the screen.
|
showNextPage
|
public void showNextPage()
Saves the current page to the
PageList by the saveCurrentPage
method and shows the next page by the showPage method.
This method is called by the ExecCommand.exec().
|
showPreviousPage
|
public void showPreviousPage()
Saves the current page to the
PageList by the saveCurrentPage method
and shows the previous page by the showPage method.
This method is called by the ExecCommand.exec().
|
insertNewPage
|
public void insertNewPage()
Inserts a new page before or after the current page.
This method is called by the ExecCommand.exec().
∙ Saves the current page to the PageList by the saveCurrentPage method.
∙ Calls the JOptionPane.showInputDialog method to inquire the user whether to
insert before the current page or after that.
∙ Creates a new PageData object with a new ContainerList (ArrayList) and a new UndoDrawManager.
∙ Adds the new PageData object to the PageList.
∙ Shows the new page by the showPage method.
|
deletePage
|
public void deletePage()
Deletes the current page.
This method is called by the ExecCommand.exec().
∙ Calls the JOptionPane.showConfirmDialog method to confirm the user for
deleting the current page. If the user's reply is "No", then returns.
∙ Deletes the current page from the PageList.
∙ If the deleted page is the last page of the PageList,
then shows the previous page, otherwise shows the next page.
If no page remains after deletion, then shows the warning message by the JOptionPane.showMessageDialog
method and inserts a new page by the insertNewPage method.
|
movePage
|
public void movePage(int selectedPage, int moveTo)
Parameter:
selectedPage - The number of the page to be moved.
moveTo - The page number which the selected page is moved to.
1≤moveTo ≤ (The number of the total pages +1)
Processing:
This method is called by the DialogOfPageLayout
class to change the pages layout - the order of the pages.
|
pageSetup
|
public void pageSetup(int sheetSize, int orientation)
Parameters:
sheetSize - The sheet size.
The sheet sizes are defined in the DrawParameters
such as DrawParameters.A4,
DrawParameters.A3 and so on.
orientation - The sheet orientation such as DrawParameters.Landscape, DrawParameters.Portrait
Processing:
This method is called by the DialogOfPageSetupAction class. Sets the parameters to the corresponding fields of the DrawParameters.
|
showPage
|
private int showPage(int selectedPage)
This method is called by the FileIO.fileOpen() and the methods of this class.
Parameters:
selectedPage - The number of the page to be shown.
1≤selectedPage ≤ The number of the total pages
Returns:
0: normal end
-1: The selected page is not found.
Processing:
∙ Retrieves the PageData object from the PageList and Retrieves the ContainerList and ViewData from the PageData.
∙ Sets the ContainerList to the ContainerManager and the ViewData to the field of DrawParameters by the ViewDataUtil.setViewData method.
|
saveCurrentPage
|
private void saveCurrentPage()
This method is called by the FileIO.save(),
FileIO.saveAs(),
DialogOfPageLayout
and the methods of this class.
Processing:
Save the PageData of the current page on display to the PageList.
Gets the ContainerList from the
ContainerManager
and the ViewData
by the ViewUtil.getViewData method,
then sets them to the PageData.
|
getContainersList
|
public ArrayList getContainerList(int page)
This method is called by the DialogOfPageLayout、
PrintableDrawPage etc.
and the methods of this class.
Returns the
ContainerList stored in the PageData object of the page
in the PageList.
|
getViewData
|
public ViewData getViewData(int page)
Returns the ViewData stored in the PageData object of the page in the PageList.
|
2. Class PageData
return=>page top
public class PageData implements Serializable
Field
|
Description
|
ContainerList
|
ArrayList ContainerList
The ArrayList object which is storing the shape objects of a page and managed
by the ContainerManager.
=>ContainerList
|
viewData
|
ViewData viewData
A ViewData object for a page which consists of a sheet size, sheet orientation,
scale factor, a left top position of view and so on.
|
undoDrawManager
|
UndoDrawManager undoDrawManager
An UndoDrawManager object for a page which stores undoable edit objects of the page.
|
Method
|
Description
|
Constractor
|
public PageData(ArrayList containerList, ViewData viewData, UndoDrawManager
undoManager)
Sets the parameters to the corresponding fields.
|
setContainerList
|
public void setContainerList(ArrayList containerList)
Sets the parameter to the ContainerList.
|
setViewData
|
public void setViewData(ViewData viewData)
Sets the parameter to the viewData.
|
getUndoDrawManager
|
public UndoDrawManager getUndoDrawManager()
Returns the undoDrawManager.
|
toString
|
public String toString()
Returns the string representing this object.
|
3. Class ViewData
return=>page top
public class ViewData implements Serializable
The ViewData object is used to manege the view data of each
page. It is set to the PageData object together with the ContainerList and UndoDrawManager objects.
Method
|
Description
|
toString
|
public String toString()
Returns the string representing this object.
|
writeViewData
(static)
|
public static int writeViewData(ObjectOutputStream out, ViewData viewData,
String version)
Writes the ViewData object to the file.
|
readViewData
(static)
|
public static ViewData readViewData(ObjectInputStream in)
Reads the ViewData object from the file.
|
4. Class ViewUtil
return=>page top
public class ViewUtil
Method
|
Description
|
zoom
(static)
|
public void zoom(double scale)
∙ Zooms in/out the shape objects on the DrawPanel.
A shape displayed at the center of the viewport keeps its position
in the viewport after zooming.
=>The light blue elliptic shape in the Figure 3
∙ The size of the DrawPanel is set by the JComponent.setPreferredSize(Dimension preferredSize) in zooming operation.
The zooming of the shape objects drawn on the DrawPanel is performed
by the g2.setScale(scale,scale) in the DrawPanel.paintBuffer(Graphics g)
and the drawing method of the ShapeContainer.drawShape(Graphics g).
Parameters:
scale - The scale factor to be set.
Processing:
∙ Calculates the point on the DrawPanel corresponding the ViewPort center after zooming by the SwingUtilities.convertPoint method.
∙ Calculates the ViewPosition of the ViewPanel using the above calculated point and sets the ViewPosition to the ViewPort.
∙ Calls the setView method.
|
getViewPanel
(static)
|
public JPanel getViewPanel(JPanel drawPanel)
Parameter:
drawPanel - The DrawPanel object.
=>Figure 2
Returns:
The panel object (ViewPanel in the Figure 2).
Processing:
If the parent of the DrawPanel
doesn't exist, then this method creates a new panel object (ViewPanel)
and adds the DrawPanel to the new panel.
If the parent of the DrawPanel exists, then returns the parent.
|
setView
(static)
|
public void setView(double scale, Point viewLeftTop)
Parameters:
scale - The scale factor
viewLeftTop - The view coordinates of the ViewPanel corresponding to the upper left corner of the viewport.
This ponts is described as the ViewPosition in the JViewport in the Java api.
=>Figure 2
Processing:
∙ Caluculates the size of the DrawPanel after zooming.
Gets the current sheet size by the
getSheetSizeByPixel method of the DrawParameters.
Determnes the size of the DrawPanel as scale*sheetSize and sets it
by the JComponent.setPreferredSize(Dimension size) method.
∙ Gets the ViewPanel by the getViewPanel method
and sets it to the viewport of the JScrollPane.
JViewport viewport=scrollPane.getViewport();
viewport.setView(viewPanel);
∙ Sets the ViewPosition of the ViewPanel to the viewport.
viewport.setViewPosition(new Point((int)viewLeftTopX,(int)viewLeftTopY));
∙ Finally calls the revalidate method of the DrawPanel.
|
moveView
(static)
|
public static void moveView(Point mousePosition)
Parameters:
mousePosition - The current mouse position.
Processing:
If the mousePosition is located outside the view port, then moves the view (substantially, it's the DrawPanel) toward the mousePosition.
The amount of the move is predefined by the constant (currently 5 pixels).
To move the view, calls the setView method with a new viewPosition.
|
getViewData
(static)
|
public static ViewData getViewData()
Returns the current view conditions by the ViewData object.
|
setViewData
(static)
|
public static void setViewData(ViewData data)
Sets the data to the current view conditions.
|
getViewPosition
(static)
|
public static Point getViewPosition()
Returns the current view position.
|
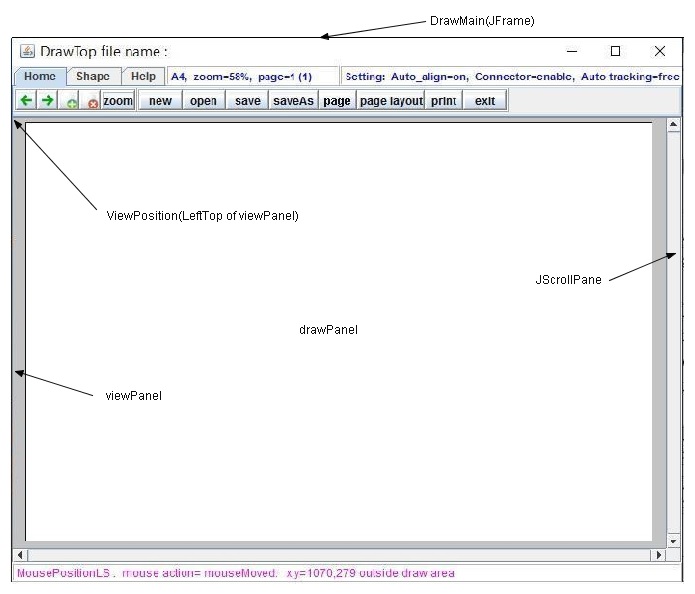
(a) Configuration of the window
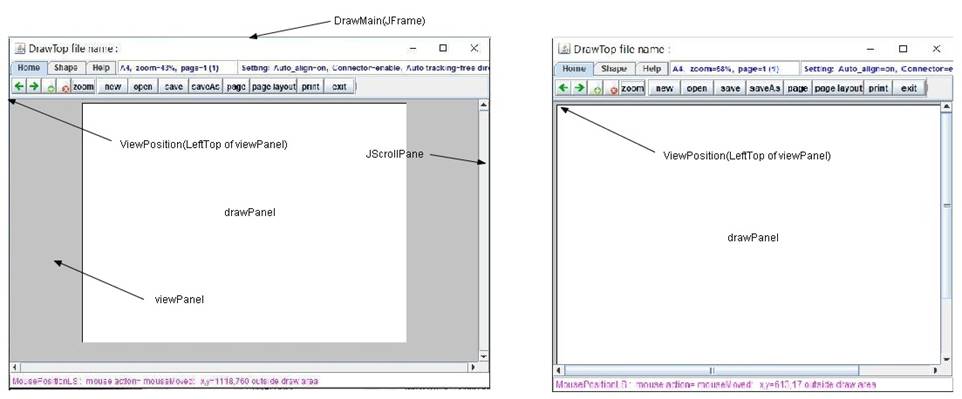
(b) Expanding the window horizontally(c) Shrinking the window
|
Figure 2 Configuration of the window
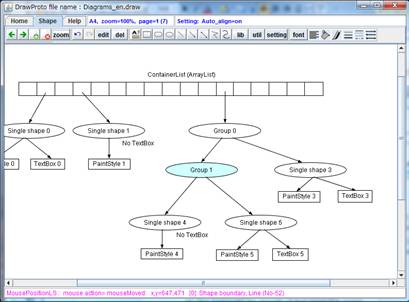
Scale factor: 100%
|
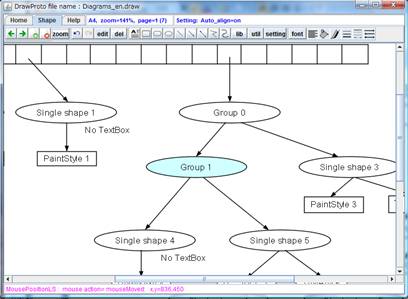
Zoom in to the scale factor of 141%
|
Figure 3 Zoom
|