1. Overview
All the drawing methods are triggered by the DrawPanel.paint method.
The DrawPanel.paint method retrieves shape objects
(ShapeContainer objects) by the
ContainerManager and calls the
ShapeContainer.drawShape method.
The ShapeContainer.drawShape method calls the
DrawShapeUtil.drawShapeElement method
and other DrawShapeUtil methods according to the state of the
ShapeContainer.
The DrawShapeUtil class is a collection of the methods
to draw a various kind of shapes and their auxiliaries.
The temporary shapes (TempShape objects) are used for
responding to a user's input and they are drawn
by the drawTempShape method.
:
To display a shape on the canvas, the ShapeContainer object
representing the shape must be added to the ContainerList by the
ContainerManager.addContainer method.
After added, the shape will be displayed by calling repaint method
of the DrawPanel.
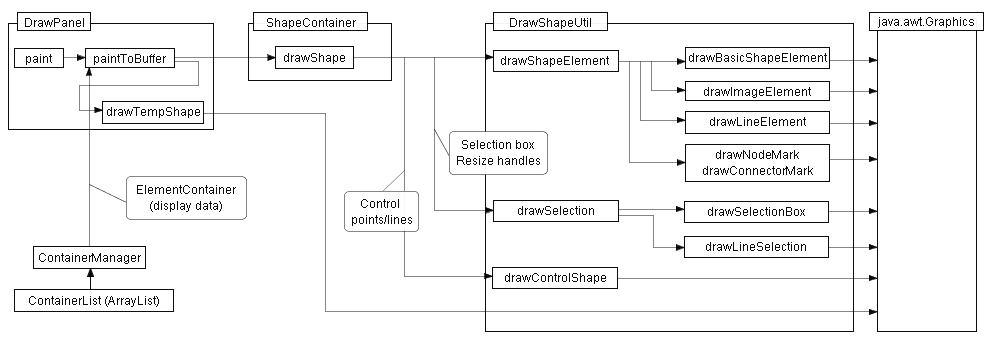
Figure 1 Diagram of drawing
=>
DrawPanel.paint,
ShapeContainer.drawShape,
DrawShapeUtil, ContainerManager,
ContainerList
2. Class DrawPanel
return=>page top
public class DrawPanel extends JPanel
Field
|
Description
|
buffer |
private Image buffer
The image buffer for double buffering.
|
tempShapeVector |
Vector tempShapeVector
Store the TempShape objects.
|
viewInformation |
String viewInformation
The string to be displayed by the showViewInformation method.
|
statusInformation |
String statusInformation
The string to be displayed by the showSettingInformation method.
|
3. Class DrawShapeUtil
return=>page top
The class is the collection of the methods to draw a shape object and its auxiliary
figures (selection box, resize handles etc.).
Method
|
Description
|
drawShapeElement
(static)
|
public static void drawShapeElement(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object.
Processing:
This method is called by the drawShape of the
ShapeContainer.
This method calls the drawStandardElement,
drawImageElement or
drawLineElement method
according to the type of the shapeElement.
In addition, this method calls the drawConnectorMark
and drawNodeMark method.
|
drawStandardElement
(static)
|
private static void drawStandardElement(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object of the basic element.
Processing:
This method is called by the drawShapeElement of this class.
Draws the shapeElement excluding LineElement, PolylineElement and ImageElement.
・Sets the clip rectangle which is slightly larger than the bounding box
of the shapeElement.
・Sets RenderingHints.VALUE_ANTIALIAS_ON by the java.awt.Graphics2D.setRenderingHint
method.
・When the shapeElement is being created, calls the drawClosedMessage method.
・If DrawParameters.DRAW_BOUNDINGBOX equals true,
then draw a bounding box which coves a shape.

Normal displayDraw bounding box
|
drawImageElement
(static)
|
private static void drawImageElement(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object of an ImageElement
Processing:
This method is called by the drawShapeElement of this class.
Draws the image (the bufferedImage field) of the ImageElement by the java.awt.Graphics.drawImage method within the bounding box
of the ImageElement.
g.drawImage(imageElement.getBufferedImage(), int)shrinkedRect.getX(), (int)shrinkedRect.getY(),
(int)shrinkedRect.getWidth(), (int)shrinkedRect.getHeight(), null);
|
drawLineElement
(static)
|
private static void drawLineElement(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object of a LineElement or PolylineElement object.
Processing:
This method is called by the drawShapeElement of this class.
Draws the line or polyline with arrow at the endpoint by calling drawLine method of this class.
・Sets RenderingHints.VALUE_ANTIALIAS_ON by the java.awt.Graphics2D.setRenderingHint
method to draw a diagonal line clearly.
・When the shapeElement is being created, calls the drawClosedMessage method.
|
drawClosedMessage
(static)
|
private static void drawClosedMessage(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object.
Processing:
Shows the string of "closed" near the center of the shapeElement, if the shapeElement is changed from a open shape to a closed shape.
|
drawLine
(static)
|
private static void drawLine(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object of a LineElement or PolylineElement object.
Processing:
・Calls the getTrimmedLine method to cut off the ends of the line or polyline.
・Calls the createArrowShape method to create a ArrowShape object.
How to draw the ArrowShape objects is specified by the
PaintSyle object linked from the
ShapeContainer of this shapeElement.
|
getTrimmedLine
(static)
|
private static Curve2D getTrimmedLine(ShapeContainer shapeContainer)
Parameters:
shapeContainer - The ShapeContainer object of a LineElement or PolylineElement object.
Processing:
If the whole line is drawn with a arrow head, it looks like Figure
(a). To avoid this, the the end of the line should be cut off slightly (Figure_(b)).
This is important especially when the line width is not small. To cut off
the end of the line, the Line2DE.getTrimmedLine method or the Polyline2DE.getTrimmedPolyline method is used.

Figure_(a)Figure_(b)
|
createArrowShape
(static)
|
private static ArrowShape createArrowShape(int position , ShapeContainer
shapeContainer)
Parameters:
position - If position equals 0, then creates a ArrowShape object at the start point of line/polyline, otherwise creates it at the
end point of line/polyline.
shapeContainer - The ShapeContainer object of a LineElement or PolylineElement object.
Returns:
The ArrowShape object.
|
drawNodeMark
(static)
|
private static void drawNodeMark(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object.
Processing:
This method is called by the drawShapeElement method and draws the segment junction points of the PolylineElement, CubicCurveElement and GeneralCurveElement.
|
drawCharacteristicMark
(static)
|
private static void drawCharacteristicMark(Graphics g, ShapeContainer shapeContainer)
This method is called from the drawShapeElement method.
Draw characteristic points output from the Curve2D.getCharacteristicPoints method.

Normal displayDraw characteristic points
|
drawConnectorMark
(static)
|
private static void drawConnectorMark(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object.
Processing:
This method is called by the drawShapeElement method and draws the identification marks if the shape element can be
a connector or the shape element allows to be connected with connectors.
|
drawSelection
(static)
|
public static void drawSelection(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object.
Processing:
This method is called by the drawShape method of the ShapeContainer to draws the selection box and resize handles (Figure 3.1, Figure 3.3).
If the shapeElement is a line, the calls drawLineSelection method, otherwise calls drawSelecetionBox method.
|
drawSelectionBox
(static)
|
private static void drawSelectionBox(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object.
Processing:
This method is called by the drawSelection method. This method draws the selection box and resize handles
(Figure 3.1) for the shapeElement and also draws square marks at the endpoints of the curve if the curve
isn't closed (Figure 3.3).
If the shapeElement is a line, the calls drawLineSelection method, otherwise calls drawSelecetionBox method.
: The line width of the selection box, the mark size of the resize handles
are independent of the scale (zoom) factor (Figure 3.2). This is because if these are dependent of the scale (zoom) factor, the
selection box and the resize handles are displayed small on the canvas
for the small scale factor, then the operation of moving a shape with its
selection box or resizing a shape with a resize handle may be difficult.
|
drawLineSelection
(static)
|
private static void drawLineSelection(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object of the LineElement object.
Processing:
This method is called by the drawSelection method to draws square marks at the endpoints of the line (Figure 3.3).
|
drawSegmentModifiers
(static)
|
public static void drawSegmentModifiers(Graphics g, ShapeContainer shapeContainer)
Parameters:
g - The java.awt.Graphics object.
shapeContainer - The ShapeContainer object.
Processing:
If the mode of the ShapeContainer linking to the ShapeElement is Command.MODIFYING_SHAPE_MODE, then this method is called. This method draws the control points and
tangent lines of the curve.
=> Figure 1.2, 1.3, 1.4 in Modifying shape
|
drawTempShape
(static)
|
public static void drawTempShape(String id, Point2D point, int size,
String message, Color color)
Parameters:
id - The identifier of a group of TempShape objects.
point - The display position of a TempShape object.
size - The size of a TempShape object. if size equals 0, the size is DrawParameters.Mark_SmallSize, otherwise DrawParameters.Mark_NormalSize.
message - The string which is displayed beside the TempShape object.
color - The color of message.
Processing:
This method used for displaying temporary and auxiliary shapes during executing
a command.
This method creates a new TempShape object and adds it to tempShapeVector by the addTempShape method of DrawPanel.
|
drawTempShape
(static)
|
public static void drawTempShape(String id, Shape shape, Stroke stroke,
Color lineColor, Color fillColor, String message, Color messageColor)
Parameters:
id - The identifier of a group of TempShape objects.
shape - The shape to be stored in a TempShape object.
stroke - The Stroke object for drawing the border of the shape.
lineColor - The Color object for drawing the border of the shape.
fillColor - The Color object for filling the shape.
message - The string which is displayed beside the TempShape object.
messageColor - The color of message.
Processing:
This method used for displaying temporary and auxiliary shapes during executing
a command.
This method creates a new TempShape object and adds it to tempShapeVector by the addTempShape method of DrawPanel.
|
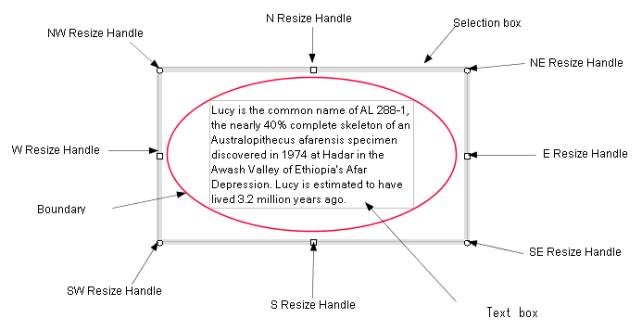
Figure 3.1 The selection box and the resize handles
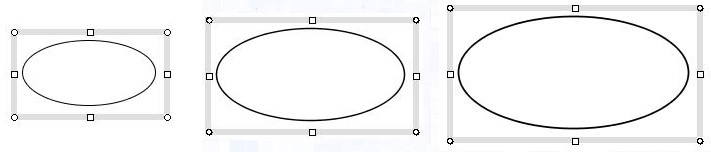 |
Scale factor=100% |
Scale factor=144% |
Scale factor=173% |
Figure 3.2 The line width of the selection box and the mark size
of the resize handles factor.
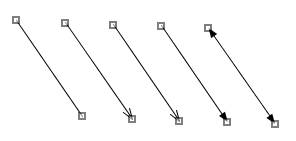
(a) Resize handles at the endpoints
|
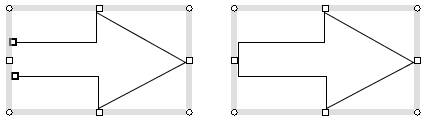
(b) Selection box and resize handles
If the shape element is not closed, draws the resize handle at the endpoints.
|
Figure 3.3
4. Class ArrowShape
return=>page top
This class represents the shape of an arrowhead.
Figure4.1 Arrow shape
Drawing the arrow line as follow.
・Cuts off the end point of a line where an arrow shape is drawn.
・Represents an arrow head by a polyline or polygon (Polyline2DE).
・Before drawing an arrow line, sets RenderingHints.VALUE_ANTIALIAS_ON
to Graphics2D.
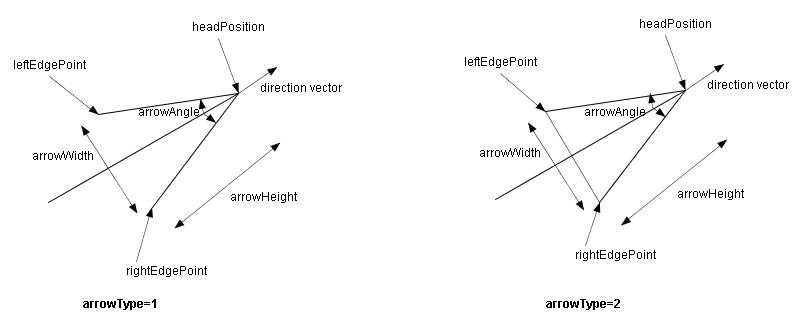
Figure 4.2 The data of an arrow head
Field
|
Description
|
ArrowAngle
|
final static double ArrowAngle
The angle of the arrow head. Currently 40 degrees.
|
MinimumArrowHeight
|
final static double MinimumArrowHeight=8d
The minimum height of the arrow head. The size of the arrow head is dependent
of the line width of an arrow line. This number prevents that the arrow head is
too small for a narrow arrow line.
|
arrowType
|
int arrowType
The type of the arrow head (1 or 2).
|
lineWidth
|
double lineWidth
The line width of the border of the arrow head.
|
headPosition
|
Vector2D headPosition
The head position of the arrow head.
|
direction
|
Vector2D direction
The direction vector of the arrow.
|
Method
|
Description
|
Constructor
|
public Arrow(int arrowType, double lineWidth, Vector2D headPosition, Vector2D
direction)
Sets the parameter to the corresponding fields.
|
getArrowShape
|
Polyline2DE getArrowShape()
Returns the Polyline2DE object representing the shape of the arrow head.
|
5. Class TempShape
return=>page top
public class TempShape
The TempShape class is used for helping a user's understanding. Auxiliary
shapes and texts of the TempShape are displayed when the system responds
to a user's input. To stop displaying, calls the clearTempShape
or clearAllTempShapes method of the DrawPanel.
Field
|
Description
|
id
|
public String id
The identification string of this object.
|
shape |
public Shape shape
The Shape object of this object. |
stroke |
public Stroke stroke
The Stroke object to draw the shape. |
lineColor |
public Color lineColor
The Color object to draw the border of the shape. |
fillColor |
public Color fillColor
The Color object to fill the shape. |
message |
public String message
The message to be displayed on the canvas. |
messageColor |
public Color messageColor
The Color object of the message. |
messageFont |
public Font messageFont
The Font object of the message. |
Method
|
Description
|
Constructor |
public TempShape(String id, Shape shape, Stroke stroke, Color lineColor,
Color fillColor, String message, Color messageColor, Font messageFont)
Sets the parameter to the corresponding field. |
getStringPosition
|
public Point2D getStringPosition()
Returns the position for drawing the message string.
|
6. Class PaintStyle
return=>page top
public class PaintStyle implements Serializable
図形の描画スタイル(輪郭線の色、塗りつぶしの色、線の太さ、線の種類、矢印など)はPaintStyleクラスで保持する。
PaintStyleオブジェクトはShapeContainerオブジェクトが作られるときにコンストラクタで一緒に作られる。
|
|
|
Figure 6.1 Line width
|
Figure 6.2 Line stroke
|
Figure 6.3 Arrow style
|
Field
|
Description
|
shapeContainer
|
ShapeContainer shapeContainer
The ShapeContainer object which has this PaintStyle object.
|
fillColor
|
public Color fillColor
The fill color of the shape element.
|
lineColor
|
public Color lineColor
The border color of the shape element.
|
lineWidth
|
public float lineWidth
The line width of the border of the shape element.
=>Figure 6.1
|
dashedStyle
|
public String dashedStyle
The String representing the line stroke of the border
of the shape element.
"solid"/"roundedDot"/"squareDot"/"dash"/"dashDot"/"dashDotDot".
=>Figure 6.2
|
dash
|
public float[] dash
The array representing the dash pattern.
The sequence of line length, space length, line length, space length ∙
・・・of the dash pattern is stored in the array.
|
arrowStyle
|
public int arrowStyle
The number representing the style of the arrow line. =>Figure 6.3
|
startArrowType
|
public int startArrowType
The type of the arrow head at the start point of the arrow line.
0: No arrow, 1: V-shaped , 2: Filled triangle.
|
endArrowType
|
public int endArrowType
The type of the arrow head at the end point of the arrow line.
0: No arrow, 1: V-shaped , 2: Filled triangle.
|
Method
|
Description
|
Constructor
|
public PaintStyle(ShapeContainer shapeContainer)
Sets the parameter to the shapeContainer.
|
getShapeContainer
|
public ShapeContainer getShapeContainer()
Returns the shapeContainer.
|
setShapeContainer
|
public void setShapeContainer(ShapeContainer container)
Sets the parameter to the shapeContainer.
|
setFillColor
|
void setFillColor(Color fillColor)
Sets the parameter to the fillColor.
|
getFillColor
|
Color getFillColor()
Returns the fillColor.
|
setLineColor
|
void setLineColor(Color lineColor)
Sets the parameter to the lineColor.
|
getLineColor
|
Color getLineColor()
Returns the lineColor.
|
setLineWidth
|
void setLineStyle(String lineWidth)
Parameters:
lineWidth - 1.0 pt/1.5 pt/2.0 pt ∙∙∙.
Processing:
Converts the parameter to integer number and sets it to
the lineWidth.
|
getLineWidth
|
float getLineWidth()
Returns the lineWidth.
|
setDashedStyle
|
public void setDashedStyle(String dashedStyle)
Parameters:
dashedStyle - "solid"/"roundedDot"/"squareDot"/"dash"/"dashDot"/"dashDotDot".
Processing:
Converts the dashedStyle to the array of integer numbers and sets it to
the dash.
|
getDashedStyle |
public String getDashedStyle()
Returns the dashedStyle. |
getLineStroke
|
public Stroke getLineStroke()
Return the Stroke object by referring lineWidth and dash.
|
setArrowStyle
|
public void setArrowStyle(String arrowStyle)
Parameters:
arrowStyle - "arrow style 1", "arrow style 2", "arrow style
3", "arrow style 4", "arrow style 5", "arrow
style 6", "arrow style 7".
Processing:
Converts thearrowStyle to integer number and sets it to the arrowStyle, startArrowType and endArrowType.
|
getArrowStyle
|
public String getArrowStyle()
Returns the arrowStyle.
|
getArrowStroke
|
public Stroke getArrowStroke()
Returns the Stroke object for drawing the arrow head by referring lineWidth.
|
getSerializable
PaintStyle
|
public SerializablePaintStyle getSerializablePaintStyle()
Returns:
The SerializablePaintStyle object.
|
setSerializable
PaintStyle
|
public void setSerializablePaintStyle(SerializablePaintStyle data)
Parameters:
data - The SerializablePaintStyle object.
Processing:
Set the contents of the data to this object.
|
getDefaultStroke
(static)
|
public static Stroke getDefaultStroke()
Returns the Stroke object whose line width is 0.5 and whose line type is
solid.
|
getDefaultStroke
(static)
|
public static Stroke getDefaultStroke(float lineWidth)
Returns the Stroke object whose line width is specified by the parameter
and whose line type is solid.
|
setPaintStyleToMenu
(static)
|
public void setPaintStyleToMenu(ShapeContainer[] shapeContainers)
Parameters:
shapeContainers - The array of the ShapeContainer objects.
Processing:
If the shapeContainers have common paint style attributes, then reflects them to the components
- button, pull down menu, color chooser or so on
- on the tool bar.
・Gets common paint style attributes of the shapeContainers(ShapeContainer).
Calls the getCommonPaintStyle method
to get the common paint style attributes. If each field in the returned PaintStyle object has a right value,
then it's a common attribute of the shapeContainers.
・Gets the components by the getMenuComponents method of the MenuUtil.
Sets each common paint attribute to the components.
: The following examples are the common attributes.
1.5 pt in Figure 6.1, "squareDot" in Figure 6.2, "arrow style 7" in Figure 6.3
|
getCommonPaintStyle
(static)
|
private static PaintStyle getCommonPaintStyle(ShapeContainer[] shapeContainers)
Parameters:
shapeContainers - The array of the ShapeContainer objects.
Processing:
If the shapeContainers objects has common paint style attributes, then sets them to a new PaintStyle
object and return the new object.
|
7. DrawParameters class
return=>page top
public class DrawParameters
This class defines the constants necessary for drawing shapes and strings.
Field
|
Description
|
Cursor
|
public static Cursor DEFAULT_CURSOR=new Cursor(Cursor.DEFAULT_CURSOR);
public static Cursor MOVE_CURSOR=new Cursor(Cursor.MOVE_CURSOR);
∙∙∙∙
public static Cursor TEXT_CURSOR=new Cursor(Cursor.TEXT_CURSOR);
The Cursor objects.
|
sheet
|
public final static int A3=0;
public final static int A4=1;
∙∙∙∙
public final static int Tabloid=8;
The integer numbers representing the sheet types.
|
sheet size |
public final static Dimension A3_Size=new Dimension(297, 420);
public final static Dimension A4_Size=new Dimension(210, 297);
∙∙∙∙
public final static Dimension Tabloid_Size=new Dimension(279, 432);
The Dimension objects representing the sheet sizes in millimeter.
|
SheetSizesMM
|
public final static Dimension[] SheetSizesMM={A3_Size, A4_Size, A5_Size,
B4_Size, B5_Size, PostCardJP_Size, Letter_Size, Legal_Size, Tabloid_Size};
The array of Dimension objects representing the sheet sizes.
|
SheetSizeString
|
public final static String[] SheetSizeString= {"A3", "A4",
"A5", "B4", "B5", "PostCardJP","Letter",
"Legal"," Tabloid"};
The array of strings representing the sheet sizes.
|
LandScape, Portrait
|
public final static int LandScape=0;
public final static int Portrait=1;
The integer numbers representing the sheet orientation.
|
SheetOrientationString
|
public final static String[] SheetOrientationString={"LandScape",
"Portrait"};
The strings representing the sheet orientation.
|
InchToMM
|
public final static double InchToMM=25.4;
Millimeter representation of one inch.
|
nchToPixels
|
public final static int InchToPixels=96;
Pixel representation of one inch.
|
Font
|
public final static Font DefaultFont=new Font(Font.DIALOG, Font.PLAIN,12);
public final static Font Font12Bold=new Font(Font.DIALOG, Font.BOLD, 12);
∙∙∙∙
public final static Font Font14Bold=new Font(Font.DIALOG, Font.BOLD, 14);
The Font objects used for drawing text on the canvas.
|
SelectionBoxOffset,
SelectionBoxWidth,
DrawAreaOffset
|
public final static double SelectionBoxOffset=6;
public final static double SelectionBoxWidth=4;
public final static int DrawAreaOffset=40;
・SelectionBoxOffset
Offset value of the selection box
from the shape bounding box.
・SelectionBoxWidth
The drawing width of a selection box.
・DrawAreaOffset:
The DrawArea means the maximum rectangle necessary for drawing a shape.
The DrawAreaOffset provides the offset amount in pixels which is used for
creating the DrawArea by offsetting the bounding box of a
selected shape
|
Mark_NormalSize,
Mark_SmallSize
|
public final static int Mark_NormalSize=6;
public final static int Mark_SmallSize=4;
・Mark_NormalSize:The mark size used for drawing resize handles etc.
・Mark_SmallSize:The smaller mark size.
|
Connection
|
public final static double ConnectionTolerance=4d;
public final static double ConnectionSmallTolerance=2d;
public final static double ClosedTolerance=3d;
public final static double Sampling_Pitch=5d;
・ConnectionTolerance
When the endpoint of a connector is moved and the distance from the endpoint
to other shape is smaller than this constant, then the connector will be
connected to the other shape.
・ClosedTolerance
If the distance between the start point and the end point of a polyline/curve
is smaller than this constant, then the polyline/curve is regarded as a
closed polyline/curve.
・Sampling_Pitch
The pitch for sampling the points on a line/polyline/curve or the border
of a closed shape.
|
DefaultAlpha, MoveAlpha
|
public final static float DefaultAlpha=0.95f;
public final static float MoveAlpha=0.6f;
・DefaultAlpha: The alpha blend in normal state.
・MoveAlpha: The alpha blend which is reffered when a shape is moved/resized.
|
DrawOnScreen,
DrawOnPrinter,
DrawOnImage
|
public final static int DrawOnScreen=0;
public final static int DrawOnPrinter=1;
public final static int DrawOnImage=2;
・DrawOnScreen:Implies drawing shapes on a computer display screen.
・DrawOnPrinter:Implies drawing shapes on a printer.
・DrawOnImage:Implies drawing shapes on the image buffer.
|
Settings
|
public static boolean AUTO_ALIGN=true;
public static boolean ENABLE_CONNECTOR=true;
public static int AUTO_TRACKING_OPTION;
public static boolean DRAW_NODE_POINTS=false;
public static boolean DRAW_TEXTLAYOUT=false;
public static boolean DRAW_BOUNDINGBOX=false;
・AUTO_ALIGN
The auto-align function is on,
if this field is true.
・ENABLE_CONNECTOR
The connector function is on, if this field is true.
・AUTO_TRACKING_OPTION
Command.FREE_DIRECTION/Command.KEEP_XY_DIRECTION_AND_POSITION
・DRAW_NODE_POINTS
Draws the marks on the segment junction points of a polyline or cubic curve, if this field is true.
・DRAW_TEXTLAYOUT
Draws the bounds of the TextLayout objects in a TextBox,
if this field is true.
・DRAW_BOUNDINGBOX
Draws the bounding box of a shape, if this field is true.
|
DrawOption |
public static int DrawMode=DrawOnScreen;
Set one of the DrawOnScreen, DrawOnPrinter or DrawOnImage to this field.
|
Method
|
Description
|
getScale |
public static double getScale()
Returns the Scale field. |
getSheetSizeByPixel |
public static Dimension getSheetSizeByPixel()
Returns the current sheet size by pixels. |
|