1. SegmentModifierreturn=>page top
The SegmentModifier object stores the segment junction points, middle points of
the segments and the tangent lines of the cubic curve segments to modify a shape.
The created objects of this class are displayed by the DrawShapeUtil.drawSegmentModifiers method.
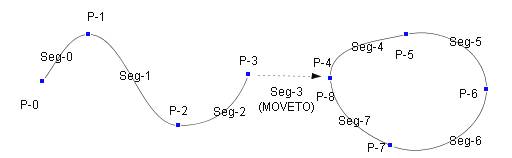
Figure 1.1 Segment number, Segment point (Junction point) number
Num. of sub path=2 |
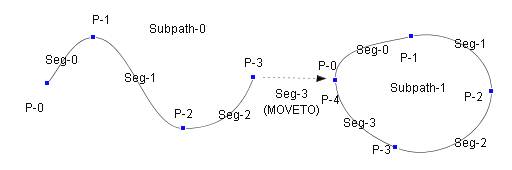
Figure 1.2 Local representation of Segment number, Segment point (Junction point) number
The segment and the segment junction point are numbered from 0 for each sub path.
This representation is used in the SegmentModifier.
|
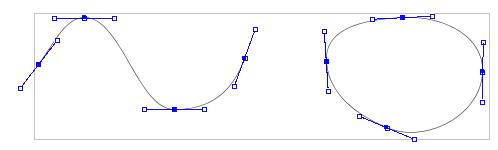
Figure 1.3 Display in the processing of the modify command
Displayed by the DrawShapeUtil.drawSegmentModifiers method.
|
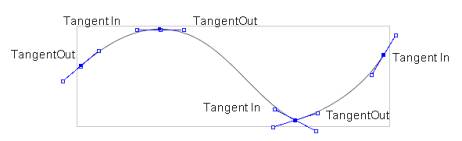
Figure 1.4 TangentIn, TangentOut
|
Field |
Description |
type
|
int type
The type of the curve segment. Sets one of the SegmentModifier.LINE/ARC/CUBIC to this field.
|
subPathIndex |
int subPathIndex
Sub path index.
|
segmentPid
|
public int segmentPid
The index of the curve segment in local representation (Figure 1.2).
|
segmentId1 |
The index of the segment located left side of the junction point specified by the segmentPid.
• For example, sets 1 to this field, If the segmentPid of this object
is 2 (see Figure 1.2).
• Sets -1, that means ineffective, to this field at the start point of
the unclosed subpath.
• Sets the last segment index (numseg) of the subpath at the start
point of the closed subpath. Here the numseg represent the number of segments in the subpath.
|
segmentId2 |
public int segmentId2
The indexof the segment located right side of the junction point specified
by the segmentPid.
• For example, sets 2 to this field, If the segmentPid of this object
is 2 (see Figure 1.2).
• Sets -1, that means ineffective, to this field at the end point of the
unclosed subpath.
• Sets the first segment index of the subpath, which is 0,
at the end point of the closed subpath.
|
segmentType1 |
public String segmentType1
Sets the type of the segmentId1 segment ("LINE"/"ARC"/"CUBIC"/"QUAD").
Sets "Null" to this field at the start point of the unclosed subpath.
|
segmentType2 |
public String segmentType2
Sets the type of the segmentId2 segment ("LINE"/"ARC"/"CUBIC"/"QUAD").
Sets "Null" to this field at the end point of the unclosed subpath.
|
p
|
Point2D p:The (x,y) coordinate of the point specified by the
segmentPid.
|
tangentIn |
Segment2D tangentIn=null
If the segment specified by the segmentId1 is "CUBIC", then sets
the tangent direction at the end point of the segment.
• Sets null to this field, at the start point of the unclosed subpath.
• Sets the tangent vector at the end point of the subpath to this field,
at the start point of the closed subpath.
|
tangentOut |
Segment2D tangentOut=null
If the segment specified by the segmentId2 is "CUBIC", then sets
the tangent direction at the start point of the segment.
• Sets null to this field, at the end point of the unclosed subpath.
• Sets the tangent vector at the start point of the subpath to this field,
at the end point of the closed subpath.
|
jointType |
int jointType=0
Sets the constant of Smooth or Cusp.
|
hitType |
int hitType=0
Sets the code of SegmentP, segmentMP, TinP1, TinP2, ToutP1 or ToutP2.
Set the above code to this field, if this object is hit in the
getHitSegmentModifier method.
|
SegmentP |
public static final int SegmentP=1;
The constant representing a junction point of segments.
|
SegmentMP |
public static final int SegmentMP=2;
The constant representing a middle point of a segment. Move this point,
then the segment is translated.
|
Tangent |
public static final int Tangent=3;
The constant representing a tangent vector at a junction point of segments.
|
Smooth |
public static final int Smooth=4;
The constant representing that the segments are connected smoothly at a junction point.
|
Cusp |
public static final int Cusp=5;
The constant representing that the segments are connected unsmoothly at a junction point.
|
TinP1 |
public static final int TinP1=6;
The constant representing that the point specified by this
is the start point of the tangentIn.
The TinP1-point is shown as a small rectangle in Figure 1.3 and 1.4.
|
TinP2 |
public static final int TinP2=7;
The constant representing that the point specified by this
is the end point of the tangentIn.
The TinP2-point is shown as a small rectangle in Figure 1.3 and 1.4.
|
ToutP1 |
public static final int ToutP1=8;
The constant representing that the point specified by this
is the start point of the tangentOut.
The TinP1-point is shown as a small rectangle in Figure 1.3 and 1.4.
|
ToutP2 |
public static final int ToutP2=9;
The constant representing that the point specified by this
is the end point of the tangentOut.
The TinP1-point is shown as a small rectangle in Figure 1.3 and 1.4.
|
codeStr |
public static String[] codeStr
The strings representing the above codes.
|
Mark_NormalSize |
final static int Mark_NormalSize=6;
The mark size.
|
Method |
Description |
Constructor
|
public SegmentModifier(int type, int subPathIndex, int segmentPid, int
segmentId1, int segmentId2, String segmentType1, String segmentType2, Point2D
p, int jointType, Segment2D tangentIn, Segment2D tangentOut)
Sets the arguments to the corresponding fields.
|
getType
|
public int getType():Returns the field variable of the type.
|
getSubPathIndex |
public int getSubPathIndex():Returns the field variable of the subPathIndex.
|
getSegmentPid
|
public int getSegmentPid():Returns the field variable of the segmentPid.
|
getSegmentId1 |
public int getSegmentId1():Returns the field variable of the segmentId1.
|
getSegmentId2 |
public int getSegmentId2():Returns the field variable of the segmentId2.
|
getP
|
public Point2D getP():Returns the field variable of the p.
|
getJointType |
public int getJointType():Returns the field variable of the jointType.
|
setJointType |
public void setJointType(int jointType):Sets the argument to the field of the jointType.
|
getTangentIn |
public Segment2D getTangentIn():Returns the field variable of the tangentIn.
|
setTangentIn |
public void setTangentIn(Segment2D tangentIn):Sets the argument to the field of the tangentIn.
|
getTangentOut |
public Segment2D getTangentOut():Returns the field variable of the tangentOut.
|
setTangentOut |
public void setTangentOut(Segment2D tangentIn):Sets the argument to the field of the tangentOut.
|
getTinP1 |
public Point2D getTinP1():Returns the start point of the tangentIn.
|
getTinP2 |
public Point2D getTinP2():Returns the end point of the tangentIn.
|
getToutP1 |
public Point2D getToutP1():Returns the start point of the tangentOut.
|
getToutP2 |
public Point2D getToutP2():Returns the start point of the tangentOut.
|
getHitType |
public int getHitType():Returns the field variable of the hitType.
|
setHitType |
protected void setHitType(int type):Sets the argument to the field of the hitType.
|
toString |
public String toString(): Returns the string representing this object.
|
clone
|
public Object clone()
Returns the clone of this object.
|
getSegmentModifiers |
public static SegmentModifier[] getSegmentModifiers(Curve2D curve2D)
Returns:
The array of the SegmentModifier objects. The returned SegmentModifier objects are created at
the junction points of the polyline and the middle points of the line segment
of the polyline.
Processing:
The creation of each SegmentModifier object at a junction point is performed
by the following getSegmentModifier method.
|
getSegmentModifier |
public static SegmentModifier getSegmentModifier(Curve2D curve2D, int type,
int subPath, int segmentPtIndex)
Parameters:
curve2D - Curve2D parametric curve that belongs to this shape element.
type - The type of the SegmentModifier. One of the types of SegmentModifier.P,
MP and Tangent is allowable.
subPath - Sub path number (index)
segmentPtIndex - Junction point number(index). See Figure 1.2.
Processing:
(1) The SegmentModifier objects at all junction points are created
for each sub path and registered with the identifier of the SegmentModifier.SegmentP.
If the sub path is closed, the segmentId1 and segmentId2 are set as follows:
• At the start point of the sub path => segmentId1=numseg-1, segmentId2=0
Here the numseg represents the number of segments of the sub path.
• At the end point of the sub path => segmentId1=numseg-1, segmentId2=0
(2) The SegmentModifier objects at middle points on the segments are
created for each sub path and registered with the identifier of the
SegmentModifier.SegmentMP.
This processing is performed for the segment types of Segment2D.LINE
and Segment2D.ARC.
(3)The SegmentModifier objects at junction points are created for each
sub path and registered with the identifier of the SegmentModifier.Tangent.
This processing is performed when at least one of the types of
the two segments located around a junction point is Segment2D.CUBIC.
|
getTangentLine |
private static Segment2D getTangentLine(Point2D point, Vector2D Tvec)
Parameters:
point - The junction point.
Tvec - The tangent vector at the junction point
Returns:
The SegmentModifier object, which represents the tangent line (vector).
Processing:
This method is called by the GeneralCurveElement.getSegmentModifiers method
and creates the Segment2D object
representing the tangent line (vector) whose start point is the point
and whose direction corresponds to the Tvec.
|
getHitSegmentModifier |
public static SegmentModifier getHitSegmentModifier(SegmentModifier[] modifiers,
Point2D point)
When this method is called, all the SegmentModifier objects are displayed
on the drawing panel by the DrawShapeUtil.drawSegmentModifiers method.
Parameters:
modifiers - All the SegmentModifier objects created by the getSegmentModifiers method.
point - The mouse position when the mouse button is pressed.
Returns:
Returns the hit SegmentModifier object when the mouse button is pressed.
Processing:
• Detection of the hit SegmentModifier object.
Compares the point parameter with the points which are given by modifiers parameter and represented by the SegmentP, SegmentMP, TinP1, TinP2, ToutP1
and ToutP2, those are shown as small rectangle in the Figure1.3
and Figure1.4. If the point is very close to a point of the modifiers[i],
then the modifiers[i] is regarded as "hit".
• Sets the hitType to the modifiers[i].
|
modify |
public static boolean modify(Curve2D curve2D, SegmentModifier hitSegmentModifier,
int ctrl, Point2D startPoint, Point2D oldPoint, Point2D currentPoint)
Parameters:
curve2D - Curve2D parametric curve which belongs to this shape element.
hitSegmentModifier - hit SegmentModifier object.
ctrl - 1 or 2 (Pressing the mouse with holding down the Shift or Ctrl key)
oldPoint - The previous point of the mouse drag.
currentPoint - The current point of the mouse drag.
Returns:
The topology of the target sub path can be changed by connecting the sub path
to another sub path at its end point, or dividing the sub path
to multiple sub paths using "Disconnect" sub-command.
If the topology of the target sub path is changed, this method returns true.
Processing:
This method is called from the GeneralCurveElement.modify method
and the GeneralCurveElement.modify method is called from the
ModifyShapeLS.mouseDragged.
The target point or shape which is moved with the mouse drag are (a) junction
point of segments, (b) middle point of a LINE or ARC segment, (c) tangent
direction of a CUBIC segment.
Which case of (a), (b) or (c) should be performed is determined by the
returned value of the hitSegmentModifier.getHitType as follows.
(a)The returned value of the hitSegmentModifier.getType is SegmentModifier.P
Moves the junction point indicated by the hitSegmentModifier using the
Segment2D.moveSegmentEndPT method.
(b)The returned value of the hitSegmentModifier.getType is SegmentModifier.MP
Moves the both end points of the segment indicated
by the hitSegmentModifier using the Segment2D.moveSegmentEndPT method.
(c)The returned value of the hitSegmentModifier.getType is SegmentModifier.TinP1,
TinP2, ToutP1 or ToutP2.
Moves the tangent direction indicated by the hitSegmentModifier using the
Segment2D.moveSegmentTangent method.
=>
Figure 1.4 in Modifying shape
|
checkDistance |
private static int[] checkDistance(Point2D P, Point2D[][] endPs)
Parameters:
P - Current mouse position.
endPs - Point2D array which is stored the end points of subpaths and is used
to calculate the minimum distance with P.
The endPs[i][0], endPs[i][1] are the start point and end point of the i - sunpath.
Returns:
Returns the (i,j) when the minimum distance to P is achieved by the endPs[i][j].
Here int[0] represents sub path index and int[1] represents
its start point or end point by 0 or 1.
|
addPoint |
public static void addPoint(Curve2D curve2D, SegmentModifier hitSegmentModifier, Point2D point)
Adds a junction point on a sub path by mouse click.
Parameters:
curve2D - Curve2D parametric curve which belongs to this shape element.
hitSegmentModifier - hit SegmentModifier object.
point - The mouse position when the mouse button was pressed.
Processing:
Gets the nearest point on the cubic curve from the point parameter by the
getShortestLine method of the
Curve2DUtil and adds the nearest point
as the segment junction point of the general curve.
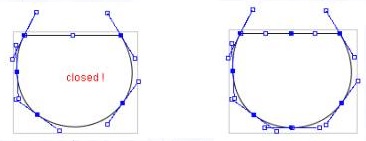 |
Original |
Adds the junction points on the upper and lower edges. |
|
deletePoint |
public static void deletePoint(Curve2D curve2D, SegmentModifier hitSegmentModifier)
Deletes the junction point on a sub path specified by a mouse click.
Parameters:
curve2D - Curve2D parametric curve that belongs to this shape element.
hitSegmentModifier - hit SegmentModifier object.
Processing:
• The the index of the junction point to be deleted is given by the hitSegmentModifier
object. Also the indices of the segments located at the both sides of
the junction point are given by the hitSegmentModifier object.
• Merge the two segments located at the both sides of
the junction point into one new segment.
Processing:
Removes the nearest segment junction point of the cubic curve from the point parameter.
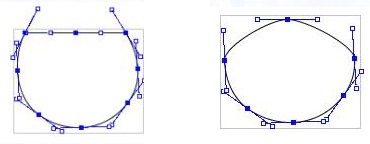 |
Original |
Deletes the two junction points on the upper edge. |
|
connectSegments |
private static Segment2D connectSegments(Segment2D segment1, Segment2D segment2)
Parameters:
segment1, segment2 - The segments to be connected.
Returns:Returns the new segment which connects the segment1 and segment2.
Processing:
Creates a new CUBIC segment which spans from the start point of the segments1
to the end point of the segment2 and which tangent direction coincides
the tangent direction of the segment1 at its start point and the tangent
direction of the segment2 at its end point.
|
smoothPoint |
public static void smoothPoint(Curve2D curve2D, SegmentModifier hitSegmentModifier, Point2D point)
Makes the sub path smooth at the junction point specified by a mouse click.
Parameters:
curve2D - Curve2D parametric curve that belongs to this shape element.
hitSegmentModifier - hit SegmentModifier object.
Processing:
The index of the junction point to be smooth is given by the hitSegmentModifier object.
Changes the tangent direction of the CUBIC segment to be smooth to the
neighboring segment at the specified junction point.
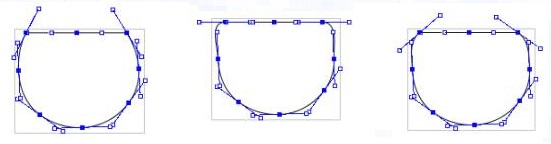 |
Original |
Makes smooth at the start and end points of the upper edge. |
Moves the tangent directions. |
|
cuspPoint |
public static void cuspPoint(Curve2D curve2D, SegmentModifier hitSegmentModifier, Point2D point)
Makes the sub path unsmooth at the junction point specified by a mouse click.
Parameters:
curve2D - Curve2D parametric curve that belongs to this shape element.
hitSegmentModifier - hit SegmentModifier object.
Processing:
Changes the tangent direction of the CUBIC segment slightly to be unsmooth to the neighboring segment.
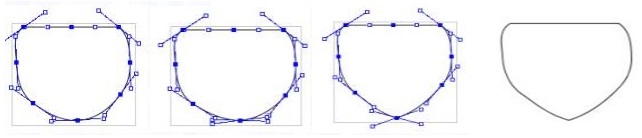 |
Original |
Changes the bottom junction point to the cusp point. |
Moves the tangent at the cusp point. |
Ordinary display. |
|
divertTangent |
private static Vector2D divertTangent(Point2D P0, Point2D P1, Point2D P2, Vector2D tangent int dir)
Parameters:
P0 - The junction point forward to P1.
P1 - The junction point specified by the mouse click.
P2 -The junction point backward to P1
tangent - tangentIn or
tangentOut at P1.
dir - The direction that the tangent direction is moved to.
If the tangent direction is moved to the side
where P1 is located for the line of P0 and P2,
then specifies 1 to the dir, if not, specifies -1 to the dir.
Returns:
Returns a new tangent which is diverted slightly from the given tangent .
|
disconnectPoint |
public static void disconnectPoint(Curve2D curve2D, SegmentModifier hitSegmentModifier, Point2D point)
Parameters:
curve2D - Curve2D parametric curve that belongs to this shape element.
hitSegmentModifier - hit SegmentModifier object.
Processing:
Sets the variables of subPath, index, index1 and index2 as follows.
int subPath=hitSegmentModifier.getSubPathIndex();
int index=hitSegmentModifier.getSegmentPid();
int index1=hitSegmentModifier.getSegmentId1();
int index2=hitSegmentModifier.getSegmentId2();

Closed path
|

Unclosed path
|
Relations between index, index1 and index2
Case |
Closed path |
Unclosed path |
index |
index1 |
index2 |
index |
index1 |
index2 |
(a)Click the start point:P-0 |
0 |
3 |
0 |
0 |
-1(=null) |
0 |
(b)Click the end point:P-4 |
4 |
3 |
0 |
4 |
3 |
-1(=null) |
(c)Click P-2 |
2 |
1 |
2 |
2 |
1 |
2 |
• Trims the segments specified by index1 and index2 slightly to disconnect the two segments.
Trimming a segment is performed by the Segment2D.trimSegment method.
• If the path is closed, the relation of the index1 and index2 is like index1>index2, so some caution is needed.
• If the path is closed and disconnects at P-1 and P-3, for example, then three sub paths are created.
Subpath-0: P-0 - P-1, Subpath-1: P-1 - P-3, Subpath-2: P-3 - P-4。
To connect the subpath-0 and subpath-2 into one subpath, this method employs
the GeneralCurve2DE.setSubPaths method.
This method uses the PathConnect object
to connect arbitrary path segments, so finally two paths are created.
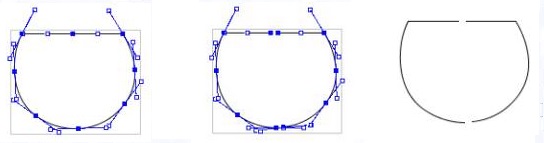 |
Original |
Disconnect at the upper and lower junction points. |
Ordinary display. |
|
2. PathConnect
return=>page top
This class is used to connect arbitrary path segments that are represented
by the segment index and the start and end points of each path segment.
Field |
Description |
segments |
PathSegment[] segments
Stores path segments.
|
jointList |
JointList jointList=new JointList()
Compares the endpoints of two path segments and if a set of their positions
is equal, then register to this list.
|
Method |
Description |
findPaths |
public PathSegment[][] findPaths(Point2D[][] endPs)
Parameters:
endPs - The start and end point of path segment. endPs[i][j] i=1,npath, j=0,1
Here i and npath represents a path index and the number
of path segments of i-path respectively, and j=0, 1 are
the indices of start point and end point.
Returns:Returns connected paths.
Substitutes the reurned value as paths[][], it means as follows:
path.length - The number of the returned paths.
path[i].length - the number of path segments of i- path.
The i - path consists of the segments of path[i][0],
path[i][1], ... path[i][path[i].length-1].
Processing:
Compares the endpoints of given path segments. If two path segments
can be connected, then connects them. Repeats this process
until all path segments are checked
|
3. PathSegment
return=>page top
This class is used in the PathConnect class.
Field |
Description |
id |
public int id
|
startP |
public Point2D startP
The start point of a path segment.
|
endP |
public Point2D endP
The end point of a path segment.
|
closed |
public boolean closed
If this path segment is closed, the sets true.
|
reversed |
public boolean reversed
If this segment is used in a connected path in reverse direction, then sets true.
|
id |
public boolean passed
If this segment is used in a connected path, then sets true.
|
4. Class ShapeElementUtilreturn=>page top
Field
|
Description
|
Limit |
final double Limit
The minimum of the width and the height of the basic shape. Currently, 5 (pixels) are set to this field.
The width and the height of the shape shouldn't be smaller than this value.
This field is referred by the resizeDiagonally and
resizeOrthogonally methods.
|
Method
|
Description
|
getBoundingBox
(public)
|
public static Rectangle2D getBoundingBox(Rectangle2D[] boxes)
Parameters:
boxes - The array of Rectangle2D objects.
Returns
The Rectangle2D object containing all the boxes.
|
getBoundingBox (public) |
public static Rectangle2D getBoundingBox(ShapeContainer[] containers)
Parameters:
containers - The array of ShapeContainer objects.
Returns
The Rectangle2D object containing all the containers.
|
getEnlargedRectangle
(public)
|
public static Rectangle2D getEnlargedRectangle(Rectangle2D box, double
wideEx, double heightEx)
Parameters:
box - TheRectangle2D object.
wideEx - The horizontal amount of expanding.
heightEx - The vertical amount of expanding.
Returns
The Enlarged Rectangle2D object.
|
getShrinkedRectangle
(public)
|
public static Rectangle2D getShrinkedRectangle(Rectangle2D rectangle,
double wideSh, double heightSh)
Parameters:
box - TheRectangle2D object.
wideSh - The horizontal amount of shrinking.
heightSh - The vertical amount of shrinking.
Returns
The shrunk Rectangle2D object. If the given box is too small to create a shrunk rectangle, returns null.
|
boxIntersectionCheck (public) |
public static boolean boxIntersectionCheck(Rectangle2D box1, Rectangle2D box2)
Parameters:
box1 - TheRectangle2D object.
box2 - TheRectangle2D object.
Returns
True if the box1 intersects with the box2.
|
getInscribedBox (public) |
public static Rectangle2D getInscribedBox(Shape shape, boolean keepAspectRatio)
Returns the rectangle inscribed in the Shape object.
Parameters:
shape - The given Shape object.
keepAspectRatio - If true, find an inscribed rectangle which is analogous to the bounding box of the given shape.
If false, find the rectangle of maximum area.
Returns
The Rectangle2D object that is inscribed in the given shape.
Processing:
(1) Create a grid covering the given shape (Figure(a)).
(2) Test whether each grid point is contained in the given shape or not
and create a bit map according to the test.
The bit map: each boolean bit corresponds to each grid point,
and if the grid point is contained in the given shape, then the bit's value is true, otherwise false.
(3) Select a center point(*) of an expected inscribed rectangle from the grid points.
(*) A center point: In Figure_(a), it is shown as a small circle mark
(4) If the keepAspectRatio is true,
calls the findSquare method, and get the inscribed rectangle shown in the Figure(c).
If the keepAspectRatio is false,
calls the findRectangle method
and get the inscribed rectangle shown in the Figure(d).
(5) Repeat (3), (4) changing the center point over the all grid points,
and we can get the inscribed rectangle of the maximum area, then return the rectangle.
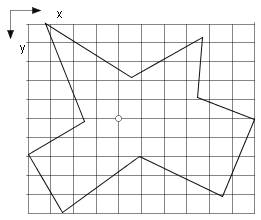 |
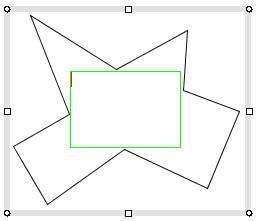 |
Figure_(a) Grid covering a given shape |
Figure_(b) Returned rectangle
(Rectangle with a green frame ) |
|
findSquare
(private) |
private static Rectangle findSquare(boolean[][] bitmap, int centerI, int centerJ)
Parameters:
bitmap - Each boolean bit in the bit map corresponds to each grid point, and
if the grid point is contained in the given shape, then the bit's value is true.
centerI - The x- directional number of the center grid point.
centerJ - The y- directional number of the center grid point.
:
The small circle mark in the figure below represents the center grid point.
Returns:
Returns the inscribed rectangle. For example,
in the figure (c) the returning rectangle is the light green rectangle.
Processing:
First, check whether the entire outer grid points around the center point
are contained in the given shape or not using the bit map.
If so, we get a rectangle contained in the given shape.
Second, check whether all the outer grid points adjacent to the rectangle
got in the previous step are contained in the given shape or not.
if so, we get a bigger rectangle contained in the given shape.
Repeat these steps, we get the maximum rectangle which is contained
in the given shape and square in the grid number space.
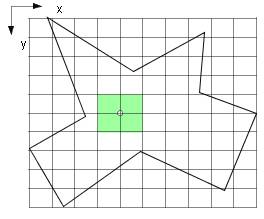 |
Figure_(c) |
|
findRectangle (private) |
private static Rectangle findRectangle(boolean[][] bitmap, int centerI, int centerJ)
Parameters:
bitmap - Each boolean bit in the bit map corresponds to each grid point, and
if the grid point is contained in the given shape, then the bit's value is true.
centerI - The x- directional number of the center grid point.
centerJ - The y- directional number of the center grid point.
:
The small circle mark in the figure below represents the center grid point.
Returns
Returns the inscribed rectangle. For example, in the figure (d) the returning rectangle is
the union of the light green rectangle, the light blue rectangle and the blue rectangles.
Processing:
First, perform the findSquare method and get the light green rectangle.
Second, test whether the light green rectangle can be extended to the left side or not.
In the figure below, the answer is "No".
Next, test whether the light green rectangle can be extended to the right side or not.
The answer is "Yes" and we get an extended rectangle
which is the union of the light green rectangle and the light blue rectangle.
Next, test whether the extended rectangle got in the previous step can
be extended to the bottom side and the topside. Then we get two extended
parts that are the blue rectangles.
Finally we get the union rectangle, which consists of the light green rectangle,
the light blue rectangle and the blue rectangles.
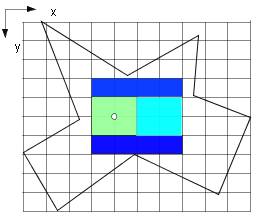 |
Figure (d) |
|
scanMap (private) |
private static boolean scanMap(boolean[][] bitmap, int startI, int endI, int startJ, int endJ)
Parameters:
bitmap - Each boolean bit in the bit map corresponds to each grid point, and
if the grid point is contained in the given shape, then the bit's value is true.
startI, endI - The x- directional numbers of the start and end.
startJ, endJ - The y- directional numbers of the start and end.
Returns
If all the boolean bits specified by the startI,
endI and startJ, endJ are true, then this method returns true.
|
resizeRectangle
(public) |
public static Rectangle2D resizeRectangle(int ctrl, Rectangle2D startBox, Point2D startPoint, Point2D newPoint, int mousePosition)
Parameters:
ctrl - If the mouse is dragged with holding down
the Shift/Ctrl/Alt key, then the value of ctrl is 1/2/3 respectively.
startBox: The bounding box which enclose the shape before resizing.
startPoint: The dragging start point.
newPoint: The dragging current point.
mouthPosition: The mouse position (code) before resizing. =>
Mouse position code
Returns: A resized Rectangle
If the ctrl equals 1 or 2, the resized rectangle is square.
If the ctrl equals 3, the resized rectangle has the same wide to height ratio as the startBox.
Processing:
This method is called by the mouseDragged method of this class and the ajustAlignment method of the AutoAlign class.
⋅ If the mousePosition equals NW_RESIZE, NE_RESIZE, SE_RESIZE or SW_RESIZE, then
this method calls the resizeDiagonally method。
⋅ If the mousePosition equals N_RESIZE, E_RESIZE, S_RESIZE, W_RESIZE, then
this method calls the resizeOrthogonally method.
:
This method creates the bounding box to which the resized shape must fits.
And updating the shape data by using the bounding box is performed by
the resize method
of the ShapeElement .
|
resizeDiagonally
(private)
|
private static Rectangle2D resizeDiagonally(int ctrl, Vector2D anchorP, Vector2D movingP, Vector2D draggedVec)
Parameters:
ctrl - See the resizeRectangle method
anchorP - The fixed point of the bounding box before resized
movingP - The moving point of the bounding box before resized
draggedVec - The vector from the dragging start point to the dragging current point.
Returns: A resized Rectangle. Same as the resizeRectangle method
Processing:
This method is called by the resizeRectangle method.
:
The width or the height of the resized bounding box is not allowed to be less than the minimum
(Limit).
Figure(b) shows the minimum height of the resized rectangle. The case of Figure(c) is not allowed.
|
resizeOrthogonally
(private) |
private static Rectangle2D resizeOrthogonally(int ctrl, int dir, Vector2D
anchorP, Vector2D movingP, Vector2D draggedVec, double fixedWH)
Parameters:
ctrl - See the resizeRectangle method
dir - The direction of resizing - 0: x-direction, 1: y-direction
anchorP - The fixed point of the bounding box before resized.
movingP - The moving point of the bounding box before resized.
draggedVec - The vector from the dragging start point to the dragging current point.
fixedWH - The width (if dir equals 1) or the height (if dir equals 0) of the bounding
box that can't be changed by resizing.
Returns: A resized Rectangle. Same as the resizeRectangle method
Processing:
: The width or the height of the resized bounding box is not allowed to be
less than the minimum (Limit). See the note of the resizeDiagonally. |
reseizeRectangle |
public static Rectangle2D reseizeRectangle(Rectangle2D rect, Rectangle2D
oldBox, Rectangle2D newBox)
Parameters:
rect - The given rectangle
oldBox - The original box
newBox - The target box
Returns:
The resized rectangle which is transformed from the rect using the transformation
from the oldBox to the newBox.
|
|