1.Class ButtonOfDebug
return=>page top
1.1 The menu and menu items of the debug command
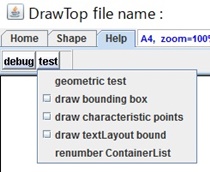 |
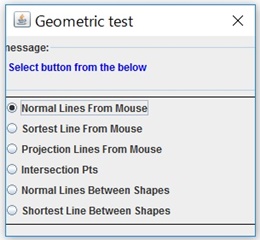 |
(a) The menu of the test command |
(b) The menu of the geometric test |
Figure 1. The test command
1.2 API
public class ButtonOfDebug extends JMenuBar implements ActionListener
:
ButtonOfDebug object is created by the DrawMenu.createDebugTestGroup
method and is added to the ToolBar panel.
Field
|
Description
|
menu
|
JMenu menu
Set JMenu object.
ButtonOfDebug is an extended class of JMenuBar and its display is the debug button in
Figure 1 (a).
The menu object is added (add) to ButtonOfDebug and displayed under the debug button
as shown in Fig. 1 (a).
|
Method
|
Description
|
Constructor
|
public ButtonOfDebug(String commandName, boolean setText, ImageIcon imageIcon,
String tip)
Creates a button with the specified ImageIcon on the button.
Parameters:
commandName - The command name.
setText - If true, displays the command name on the button.
imageIcon - The imageIcon to be displayed on the button.
tip - The text to be displayed in a tool tip.
|
setStandardButton Style
|
public void setStandardButtonStyle()
Calls the following methods.
this.menu.setFont(MenuConstants.MenuFont);
Border raisedBorder = new BevelBorder(BevelBorder.RAISED);
this.menu.setBorder(raisedBorder);
this.menu.setIconTextGap(0);
this.menu.setOpaque(true);
Color backGround=new Color(0xDDE8F3);
this.menu.setBackground(backGround);
this.menu.setForeground(Color.black);
|
setSelected |
public void setSelected(boolean selected)
Sets the parameter to the menu.
|
isSelected
|
public boolean isSelected()
Returns if the menu is selected.
|
createDebugButton (static) |
public static ButtonOfDebug createDebugButton(int width)
Parameters:
width - The width of the button.
Processing:
The settings are the follow.
ButtonOfDebug menuButton=new ButtonOfDebug("debug", true, null, commandName);
String[] menuItemNames={"System.out dialog","print menulist"};
|
actionPerformed
|
public void actionPerformed(ActionEvent e)
Executes the processing according to the menu item.
(1)geometric test menu item
Create GeometricTest object and call
GeometricTest.showDialog method.
Display Examples => Figure 2
(2)draw bounding box menu item
Set true to DrawParameters.DRAW_BOUNDINGBOX.
DrawShapeUtil.drawStandardElement and
DrawShapeUtil.drawLineElement methods
displays bounding boxes
(3)draw characteristic points menu item
Set true to DrawParameters.DRAW_CHARACTERISTIC_POINTS.
DrawShapeUtil.drawCharacteristicMark method
displays characteristic points.
(4)draw textLayout bound menu item
Set true to DrawParameters.DRAW_TEXTLAYOUT.
TextBox.drawText method displays textLayout bounds.
(5)renumber ContainerList menu item
Renumber the serial number of shapeId
which is the identifier of ShapeContainer.
Renumbering is executed in the ContainerManager.ContainerList
by ContainerManager.renumberContainerList method.
|
2 Class GeometricTest
return=>page top
2.1 Test
Geometric test checks the stability of the numerical calculations
such as the bisection method and the Newton-Raphson method(Figures below).
(a)Normal Lines From Mouse: Calculate the normal lines from the mouse
pointer to the selected shape and display the normal lines
and their end points as Figure_(a).
(b)Shortest Line From Mouse: Calculate the shortest line from the mouse pointer to
the selected shape as Figure_(b).
(c)Projection Lines From Mouse: Calculate the projection lines in 8 directions from the mouse pointer to
the selected shape as Figure_(c).
(d)Intersection Pts: Move the selected shape so that it overlaps another shape,
then calculate and display the intersection points between the two shapes as Figure_(d).
(e)Normal Lines Between Shapes: Move the selected shape to other shape,
then calculate and display the common normal lines between two shapes.(Figure_(e))
(f)Shortest Line Between Shapes: Move the selected shape to other shape,
then calculate and display the shortest line between two shapes.(Figure_(f))
∙ The multiple shortest lines are displayed for each element of a group shape.
∙ Line colors
Red line: Common normal line
Blue line: Connecting to a segment junction point(endpoint or cusp point) at least one shape.
2.2 API
public class GeometricTest
Field
|
Description
|
geometricTestLS
|
GeometricTestLS geometricTestLS
The GeometricTestLS object.
|
messageLabel
|
JLabel messageLabel
The message label on which the showMessage method outputs a message.
|
NormalLinesFromMouseButton
|
JRadioButton NormalLinesFromMouseButton
The button indicating the calculation of Figure_(a)).
|
ShortestLineFromMouseButton
|
JRadioButton ShortestLineFromMouseButton
The button indicating the calculation of Figure_(b).
|
ProjectionLinesFromMouseButton
|
JRadioButton ProjectionLinesFromMouseButton
The button indicating the calculation of Figure_(c).
|
IntersectionPtsButton
|
JRadioButton IntersectionPtsButton
The button indicating the calculation of Figure_(d).
|
NormalLinesBetweenShapesButton
|
JRadioButton NormalLinesBetweenShapesButton
The button indicating the calculation of Figure_(e)。
|
ShortestLineBetweenShapesButton
|
JRadioButton ShortestLineBetweenShapesButton
The button indicating the calculation of Figure_(f)。
|
Method
|
Description
|
Constructor
|
public GeometricTest()
Sets the following.
super(ObjectTable.getDrawMain(), "Geometric test");
this.geometricTestLS=new GeometricTestLS();
this.setName("Geometric test");
|
showDialog
|
public void showDialog()
If this dialog is already opened, this method does nothing.
Whether the dialog is opened or not can be checked by the by the
getMenuComponent of the
menuUtil.
∙ Sets the GeometricTestAction to this object as a window listener.
∙ Calls the start method of the GeometricTestLS to start the mouse listeners.
∙ Sets the buttons and the message label to this object.
∙ Shows the dialog by calling this.setVisible(true).
∙ Registers this object to MenuComponentList
by the setMenuComponent.
|
showMessage
|
protected void showMessage(String message)
Shows the message on the messageLabel.
|
getGeometricTestLS
|
protected GeometricTestLS getGeometricTestLS()
Returns the geometricTestLS.
|
3. Class GeometricTestAction
return=>page top
class GeometricTestAction extends AbstractAction implements WindowListener
4. Class GeometricTestLS
return=>page top
public class GeometricTestLS implements MouseListener, MouseMotionListener
Field
|
Description
|
mode
|
int mode
The operation mode of this object.
|
NormalLinesFromMouse_Mode |
public static int NormalLinesFromMouse_Mode=1;
The mode representing the calculation of Figure_(a). |
ShortestLineFromMouse_Mode |
public static int ShortestLineFromMouse_Mode=2;
The mode representing the calculation of Figure_(b) |
ProjectionLinesFromMouse_Mode |
public static int ProjectionLinesFromMouse_Mode=3;
The mode representing the calculation of Figure_(c) |
IntersectionPts_Mode |
public static int IntersectionPts_Mode=4;
The mode representing the calculation of Figure_(d) |
NormalLinesBetweenShapes_Mode |
public static int NormalLinesBetweenShapes_Mode=5;
The mode representing the calculation of Figure_(e) |
ShortestLineBetweenShapes_Mode |
public static int ShortestLineBetweenShapes_Mode=6;
The mode representing the calculation of Figure_(f) |
Method
|
Description
|
start
|
public void start()
This method is called by the showDialog
method of the GeometricTest and registers this object to the ListenerPanel
as a mouse listener.
|
end
|
public void end()
This method is called by the closeDialog
method of the GeometricTestAction
and removes this object from the ListenerPanel.
|
setMode
|
public void setMode(int mode)
Sets the parameter to the mode.
|
mousePressed
|
public void mousePressed(MouseEvent e)
If the mode equals IntersectionPts_Mode,
then this method calls the drawIntersectionPts method.
|
mouseDragged
|
public void mouseDragged(MouseEvent e)
If the mode equals IntersectionPts_Mode,
then this method calls the drawIntersectionPts method.
|
mouseReleased
|
public void mouseReleased(MouseEvent e)
If the mode equals GIntersectionPts_Mode,
then this method calls the drawIntersectionPts method.
|
mouseClicked
|
public void mouseClicked(MouseEvent e)
Nothing is done.
|
mouseMoved
|
public void mouseMoved(MouseEvent e)
∙ If the mode equals NormalLinesFromMouse_Mode,
then this method calls the drawNormalLines method.
∙ If the mode equals ShortestLineFromMouse_Mode,
then this method calls the drawShortestLine method.
|
mouseEntered
|
public void mouseEntered(MouseEvent e)
Nothing is done.
|
mouseExited
|
public void mouseExited(MouseEvent e)
Nothing is done.
|
drawNormalLines
|
protected void drawNormalLines(Point2D point)
∙ Gets all the selected shapes
by the getAllSigleShapeContainers
method of the ContainerManager.
∙ Calculates the normal lines from the current mouse position to the selected
shapes by the Curve2DUtil.getNormalLines method.
∙ Draws the normal lines and their end points on the boundaries of the
selected shapes by the DrawShapeUtil.drawTempShape method.
=>Figure (a)
|
drawShortestLine
|
protected void drawShortestLine(Point2D point)
∙ Gets all the selected shapes
by the getAllSigleShapeContainers
method of the ContainerManager.
∙ Calculates the shortest lines from the current mouse position to
the selected shapes by the Curve2DUtil.getShortestLine method.
∙ Draws the shortest lines and their end points on the boundaries
of the selected shapes by the DrawShapeUtil.drawTempShape method.
=>Figure (b)
|
drawProjectionLines
|
protected void drawProjectionLines(Point2D point)
Parameters:
point - The position of the mouse cursor
Processing:
∙ Get all of the selected single shapes
by the ContainerManager.getAllSigleShapeContainers method.
∙ From the current mouse position (point), calculate the projection lines to
the selected single shapes on 8 directions with 45-degree pitch by the
Curve2DUtil.getProjectionLines method.
∙ Display marks at the position of the projection point on the shape,
and display the projection lines by the DrawShapeUtil.drawTempShape method.
=>Figure (c)
|
drawIntersectionPts
|
protected void drawIntersectionPts()
∙ Calculates the intersection points between the selected shapes and the other shapes
by the Curve2DUtil.getIntersectionPts method.
∙ Draws the intersection points by the DrawShapeUtil.drawTempShape method.
=>Figure (d)
|
drawNormalLinesBetweenShapes
|
protected void drawNormalLinesBetweenShapes()
∙ Get the selected shape and its nearest shape(target shape)
by the getClosestShapes method.
∙ For each selected shape and target shape,
get the single shape elements respectively.
Considering also that the shape is a group shape, get the single shape elements of the selected shape
and the target shape as arrays by the
ShapeContainer.getGroupedSingleShapeContainers method.
∙ Calculation of common normal lines.
Calculate common normal lines for combinations of the single element shapes
of the selected shape and those of the target shape by the
getNormalLinesBetweenClosedCouple of this class.
∙ Display results
Create the display lines of the calculation result based on the returned
Curve2DUtil.NormalsInfo objects
and display them and marks if there is intersection points
by the the DrawShapeUtil.drawTempShape.
=>Figure (e)
|
getClosestShapes
|
protected ShapeContainer[] getClosestShapes()
Returns:
Return the selected shape to the ShapeContainer[0]
and return a shape nearest to the the selected shape
which is denoted by a target shape to the ShapeContainer[1].
The shapes to be returned may be a group shape.
Processing:
∙ Get the selected shape by the
ContainerManager.getSelectedContainers method.
∙ Get the target shape
Get the all of the shapes stored in the ContainerManager.ContainrtList
by the ContainerManager.getContainers method.
Cover each of them by a rectangle and caluculate the distance between the rectangle covering the selected shape and other rectangles
by the Curve2DUtil.distanceBetweenBoxes,
then the nearest shape to the selected shape can be acquired as the target shape.
|
getNormalLinesBetweenClosedCouple
|
protected Vector getNormalLinesBetweenClosedCouple(ShapeContainer selectedShape, ShapeContainer targetShape)
Parameters:
selectedShape - The selected shape.
targetShape - The closest shape to the selected shape. This object is denoted by target shape<.BR>
Processing:
∙ For each selected shape and target shape,
get the single shape elements respectively.
Considering also that the shape is a group shape, get the single shape elements of the selected shape
and the target shape as arrays by the
ShapeContainer.getGroupedSingleShapeContainers method.
∙ Calculation of common normal lines.
Calculate common normal lines for combinations of the single element shapes
of the selected shape and those of the target shape by the
Curve2DUtil.getNormalLinesBetweenShapes.
The output data of the above method is a Vector object and stores
Curve2DUtil.NormalsInfo objects.
Finally this method moves the Curve2DUtil.NormalsInfo objects
to in another Vector object (normalLines) and returns the normalLines.
|
drawShortestLinesBetweenShapes
|
protected void drawShortestLinesBetweenShapes()
Processing:
∙ Get the selected shape and its nearest shape(target shape)
by the getClosestShapes method.
∙ For each selected shape and target shape,
get the single shape elements respectively.
Considering also that the shape is a group shape, get the single shape elements of the selected shape
and the target shape as arrays by the
ShapeContainer.getGroupedSingleShapeContainers method.
∙ Calculation of common normal lines.
Calculate common normal lines for combinations of the single element shapes
of the selected shape and those of the target shape by the
Curve2DUtil.getShortestLinesBetweenClosedCouple of this class.
∙ Display results
Create the display lines of the calculation result based on the returned
Curve2DUtil.NormalsInfo objects
and display them and marks if there is intersection points
by the the DrawShapeUtil.drawTempShape.
=>Figure (f)
|
getShortestLinesBetweenClosedCouple
|
protected Vector getShortestLinesBetweenClosedCouple(ShapeContainer selectedShape,
ShapeContainer targetShape)
Parameters:
selectedShape - The selected shape.
targetShape - The closest shape to the selected shape. This object is denoted by target shape.
Processing:
∙ For each selected shape and target shape,
get the single shape elements respectively.
Considering also that the shape is a group shape, get the single shape elements of the selected shape
and the target shape as arrays by the
ShapeContainer.getGroupedSingleShapeContainers method.
∙ Calculation of common normal lines.
Calculate common normal lines for combinations of the single element shapes
of the selected shape and those of the target shape by the
Curve2DUtil.getShortestLineBetweenShapes.
The output data of the above method is a Vector object and stores
Curve2DUtil.NormalsInfo objects.
Finally this method moves the Curve2DUtil.NormalsInfo objects
to in another Vector object (shortestLines) and returns the shortestLines.
|
|