1. Overview
(1) SerializableElement
The SerializableElement class is the serializable class which contains all the fields of the ShapeContainer, ShapeElement, TextBox and PaintStyle objects. So the ShapeContainer object can be inverted from the SerializableElement object(s), even if it represents a group of shapes.
The SerializableElement can be written/read to/from a file and is implemented with the write/read
methods.
(2) Related classes
The serializable classes for the Curve2D,
Segment2D and java.text.AttributedString classes are defined as the
SerializableCurve2D,
SerializableSegment2D and
SerializableAttributedString.
(3) Utilizing in other functions
Because the SerializableElement object and the related objects are compact and handy, so these are utilized
in the following functions.
∙ Editing shape/text (cut/copy and caste)
Shape objects and text objects are converted to the
SerializableElement object and
the related objects, then they are copied to the clipboard.
∙ Undo, Redo
The SerializableElement object
and the related objects are set to the
UndoableDrawEdit object.
2. Class SerializableElement
戻る=>page top
public class SerializableElementimplements Serializable
The SerializableElement class is the class which contains all the fileds
of the ShapeContainer, ShapeElement, TextBox and PaintStyle objects and has only a few simple methods like toString.
If the ShapeContainer represents a single shape, it is easy to convert to SerializableElement.
However, if the ShapeContainer represents a group shape, the group SerializableElement
is represented by the tree structure as shown in Figure 2.1 (e). To do this, the child SerializableElements are stored in the field variable:
chirdrenList (ArrayList) of the parent SerializableElement.
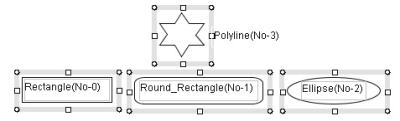
(a)Original |
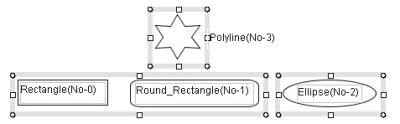
(b)Grouping two shapes. |
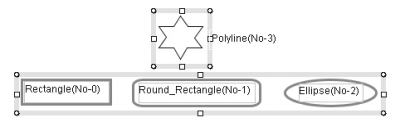
(c)Grouping the group shape and the elliptic shape. |
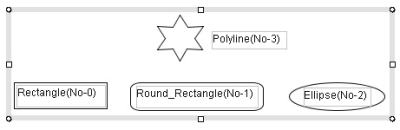
(d)Grouping the group shape and the star shape. |
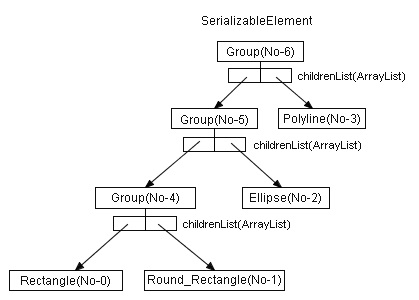
(e)The tree structure of the above group (d) |
Figure 2.1 SerializableElement of a group shape
Method
|
Description
|
getGroupedElements |
public SerializableElement[] getGroupedElements()
Returns:
If this SerializableElement object represents a single shape, returns itself. If it represents a group shape, returns itself and all its chilld SerializableElement objects.
For example, returns the array of the Group(No-6), Group(No-5), Group(No-4),
Rectangle(No-0), Round_Rectangle(No-1), and Ellipse(No-2) for the group
shape in Figur 2.1 (d) and (e)
Processing:
Calls the next getGroupedElementsE method.
|
getGroupedElementsE |
public void getGroupedElementsE(Vector vector)
Parameters:
vector - Vector which stores the child SerializableElement objects.
Processing:
Calls this method recursively to search the tree of the group shape and return all the child SerializableElement objects which are stored in the vector.
|
getGroupTreeDepth |
public int getGroupTreeDepth(String shapeId)
This method is used to indent the print out of a child SerializableElement object.
=>
Figure 2.2
Parameters:
shapeId - The identifier of a shape object.
Returns:
Returns the tree depth of the child SerializableElement object which coincides with shapeId.
For example, the tree depth of the Group(No-6) is 0 and that of the Group(No-4) is 2 in Figure 2.1 (e).
Processing:
Calls the next getGroupTreeDepthE method.
|
getGroupTreeDepthE |
public void getGroupTreeDepthE(Vector vector)
Parameters:
vector - Stores shapeId(String), treeDepth(Integer) and findDepth(Integer) to the vector.
∙ treeDepth - The tree depth where this method is searching(work variable).
∙ findDepth - The tree depth of the child SerializableElement object which coincides with shapeId.
If this method can't find such a child SerializableElement object , sets -1 to the findDepth and returns.
Processing:
Calls this method recursively to search the tree of the group
shape such as Figur 2.1 (e).
|
clone |
public Object clone()
Returns the clone of this object.
|
cloneHashMap |
private HashMap<String, Object> cloneHashMap(HashMap<String, Object> hashMap)
Returns the clone of HashMap filed in this object.
|
toString
|
public String toString()
Returns a string representation of this object.
Determines the number of spaces in indentation by the treeDepth value
returned from the getGroupTreeDepth method
and the string of the header, then calls the next toStringE method.
=>Figure 2.2
|
toStringE |
private String toStringE(int indent, String header)
Returns a string representation of of this object.
|
toShortString
|
public String toShortString()
Returns a short string representing this object.
The indentaion of this method is as same as the toString.
Calls the next toShortStringE method.
=>Figure 2.2
|
toShortStringE |
private String toShortStringE(int indent, String header)
Returns a short string representation of this object.
|
toVeryShortString
|
public String toVeryShortString()
Returns a very short string representing this object.
The indentaion of this method is as same as the toString.
Calls the next toVeryShortStringE method.
=>
Figure 2.2
|
toVeryShortStringE |
private String toVeryShortStringE(int indent, String header)
Returns a very short string representation of this object.
|
3. Class SerializableCurve2D
戻る=>page top
public class SerializableCurve2D implements Serializable
This class provides the serializable class which has all the fileds of
the Curve2D and the methods to write/read to/from the file. This object is created
by the getSerializableCurve2D method of
the Curve2D and set to the Curve2D object
by the setSerializableCurve2D method.
Field
|
Description
|
type
|
public int type
The returned value of the getType method of the Curve2D. Curve2D.RECTANGLE/ROUND_RECTANGLE/ELLIPSE/LINE...etc.
|
doubleData
|
public double[] doubleData
The double array which stores the characteristic data of the parametric
curve. Currently this field isn't referred.
|
points
|
public Point2D[] points
The array which stores the characteristic points of the parametric curve.
Currently this field isn't referred.
|
serializableSegments
|
public SerializableSegment2D[] serializableSegments
The array storing the SerializableSegment2D objects which compose the parametric curves of this object.
|
versions
|
public static final String[] versions
The array of the String objects representing versions of this class.
|
4. Class SerializableSegment2D
戻る=>page top
public class SerializableSegment2D implements Serializable
This class provides the serializable class which has all the fileds of
the Segment2D and the methods to write/read to/from the file.
This object is created
by the getSerializableSegment2D method
of the Segment2D and set to the
Segment2D object
in the setSerializableCurve2D method.
Field
|
Description
|
type
|
public int type
The type field of the Segment2D.
|
shape
|
public Shape shape
The shape field of the Segment2D.
|
affineTransform
|
public AffineTransform affineTransform
The affineTransform field of the Segment2D.
|
versions
|
public static final String[] versions
The array of the String objects representing versions of this class.
|
Method
|
Description
|
writeSerializable
Segment2D
|
public static int writeSerializableSegment2D(ObjectOutputStream out, SerializableSegment2D
sSegment2D) throws Exception
Parameters:
out - The ObjectOutputStream object.
sSegment2D - The SerializableSegment2D object.
Processing:
∙ Writes the version to the file.
∙ Writes each field of the SerializableSegment2D object to the file.
|
readSerializable
Segment2D
|
public static SerializableSegment2D readSerializableSegment2D(ObjectInputStream
in) throws Exception
Parameters:
in - The ObjectInputStream object.
Returns:
The SerializableSegment2D object.
Processing:
∙ Reads the version to the file.
∙ Reads each field of the SerializableSegment2D object from the file.
|
5. Class SerializableAttributedString
戻る=>page top
public class SerializableAttributedString implements Serializable, Cloneable
This class provides the serializable class representing the java.text.AttributedString.
This object is represented by the String object and the AttributedInterval object just like the AttributedStringUtil.
This object is created by the getSerializableAttributedString method of the CommittedTextContainer and set to the CommittedTextContainer object in the setSerializableAttributedString method.
Field
|
Description
|
string
|
private String string
Sets the string (plain text) of the AttributedString object.
|
attributedIntervalList
|
public ArrayList attributedIntervalList
The ArrayList storing the AttributedInterval object.
Just same as the arrayList of the AttributedStringUtil.
|
versions
|
public static final String[] versions
The array of the String objects representing versions of this class.
|
Method
|
Description
|
Constructor
|
public SerializableAttributedString(AttributedCharacterIterator iterator)
Creates a new AttributedStringUtil object and gets the string and the attributedIntervalList and sets them
to the correnponding fields.
|
getString
|
public String getString()
Returns the string.
|
setString
|
public void setString(String str)
Sets the parameter the string.
|
getAttributedIntervalList
|
public ArrayList getAttributedIntervalList()
Returns the attributedIntervalList.
|
setAttributedIntervalList
|
public void setAttributedIntervalList(ArrayList list)
Sets the parameter to the attributedIntervalList.
|
getAttributedString
|
public AttributedString getAttributedString()
Returns the AttributedString object.
|
clone
|
public Object clone()
Returns the clone object.
|
toString
|
public String toString()
Returns the string representing this object.
|
writeSerializable
AttributedString
(static)
|
public static int writeSerializableAttributedString(ObjectOutputStream
out, SerializableAttributedString sAttributedString) throws Exception
Parameters:
out - The ObjectOutputStream object.
sAttributedString - The SerializableAttributedString object.
Processing:
∙ Writes the version to the file.
∙ Writes each field of the SerializableAttributedString object to the file.
|
readSerializable
AttributedString
(static)
|
public static SerializableAttributedString readSerializableAttributedString(ObjectInputStream
in) throws Exception
Parameters:
out - The ObjectOutputStream object.
Returns:
Returns SerializableAttributedString object.
Processing:
∙ Reads the version to the file.
∙ Reads each field of the SerializableAttributedString object from the file.
|
6. SerializableTextBox
戻る=>page top
public class SerializableTextBox implements Serializable
This class isn't used for writing to the file but used for setting an undo/redo
object in the UndoDrawableEdit.ChangeTextBox object.
Method
|
Description
|
toString
|
public String toString()
Returns the string representing this object.
|
7. SerializablePaintStyle
戻る=>page top
public class SerializablePaintStyle implements Serializable
This class isn't used for writing to the file but used for setting an undo/redo
object in the UndoDrawableEdit.ChangePaintStyle object.
Method
|
Description
|
toString
|
public String toString()
Returns the string representing this object.
|
8. Class SerializableElementUtil
戻る=>page top
public class SerializableElementUtil
This class provide the conversion/inversion methods between a
ShapeContainer object
and a SerializableElement object.
This class is used for the followings.
∙ Editing shape/text (cut/copy and caste)
∙ Undo, Redo
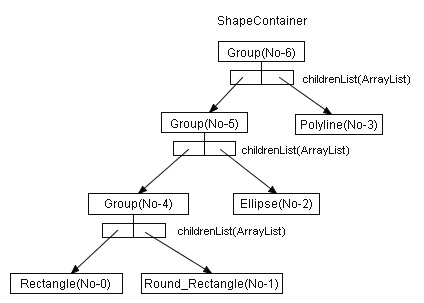
(a) ShapeContainer objects of a group
|
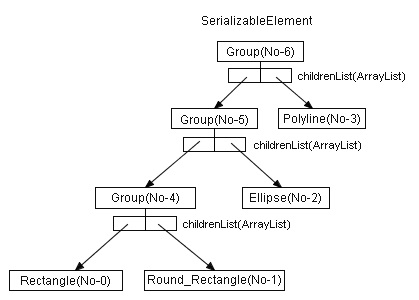
(b) The SerializableElement objects
converted from the ShapeContainer of a group |
|
Figure 8.1 The data format of a group of the ShapeContainer and SerializableElement
|
Field
|
Description
|
versions
|
public static final String[] versions
The array of the String objects representing versions of this class.
|
|